Use Cases and Examples
Bash printf prints text on a terminal with multiple formatting options. It’s probably most useful to print in a shell script, but can be used on the command line as well.
$ name = Sam
$ printf "Hi $name"
Hi Sam
Run in Warp
In cases where we want more control over how content is displayed, we can leverage the additional formatting specifiers and arguments to fit our needs.
printf syntax
printf [-v var] [format specifiers] [arguments]
var: optional and if used, the output will not be printed but will be assigned to the variable. This is not to be confused with printing variables which you can do by prefixing the variable with $.
# This first command stores the content as a variable and will not output anything
$ printf -v fooBar "Welcome"
$ printf "$fooBar"
"Welcome"
Run in Warp
format specifiers: string(s) that may contain one or more of:
- Normal characters
- Backslash-escaped characters
- \b - backspace character
- \n - newline character
- \t - horizontal tab space
- \v - vertical tab space
- \” - quotation character
- \\ - backslash character
$ printf "Hellow\\b \\"John\\\\Joe\\"\\n"
Hello "John\\Joe"
Run in Warp
Conversion specifications (e.g. to format string or format number)
- %s - to treat as a string
- %c - to treat as a single character
- %f - to treat as a floating number
- %d - to treat as a signed integer
- %u - to treat as an unsigned integer
- %% - to print the percentage symbol
- %X - to print with X character wide widths specified
- %.X - to print with a precision modifier of X characters
- %- - to print left-justified. note: by default it is right-justified.
$ printf "%s are %d%% off! OM%c!" "Shirts" 5 "GGGGG"
"Shirts are 5% off! OMG!"
$ printf "%5s: %f\\n" "Abe" 3.5 "John" 5 "It" 8.221
Abe: 3.500000
John: 5.000000
It: 8.221000
$ printf "%-5s: %f\\n" "Abe" 3.5 "John" 5 "It" 8.221
Abe : 3.500000
John : 5.000000
It : 8.221000
Run in Warp
arguments: can be any number of values and/or variables. In the case that there are more arguments than format specifiers, then the arguments will get reused.
# number of arguments exceeds format specifiers
$ printf "One %d Two %d Three %d\\n" 1 2 3 4
One 1 Two 2 Three 3
One 4 Two 0 Three 0
# number of arguments is fewer than format specifiers
$ printf "One %d Two %d Three %d\\n" 1 2
One 1 Two 2 Three 0
Run in Warp
Tip: If you are on zsh and you may be seeing a % at the end of each line. This is zsh's way of noting that the preceding command output was a partial line. You can disable it by updating the PROMPT_EOL_MARK configuration within your ~/.zshrc file.
printf makes it easy for engineers to output readable content
Being able to use printf for all of its functionalities helps us as engineers understand our code easier and in a human-friendly way. For example, imagine you were trying to debug a script with the following variables.
USD=1.0
CNY=7.188832
BZD=55.442
TWD=31.7
Run in Warp
In the example below, we don’t leverage the variable printing that printf has, so we end up hardcoding the currency amounts. Additionally, it is harder to read because it is all on one line, and all of the variables have different precisions.
$ printf "Current Currency Rates USD: 1 / CNY: 7.188832 / BZD: 0.55 / TWD: 31.7"
Current Currency Rates USD: 1 / CNY: 7.188832 / BZD: 0.55 / TWD: 31.7
Run in Warp
Instead, if we utilize the capabilities of printf we can write legible statements that can be modified and built on top of. Below, we are printing the variables and formatting the output with the width specified and float precision.
$ printf "Current Currency Rates\\n"
$ printf "%4s: %.2f\\n" "USD" $USD "CNY" $CNY "BZD" $BZD "TWD" $TWD
Current Currency Rates
USD: 1.00
CNY: 7.19
BZD: 0.55
TWD: 31.70
Run in Warp
echo vs printf
When writing scripts, echo is the simplest command to print to the standard output. By default, it adds a new line so that you don’t need to! You can print strings and variables and add options to escape backslashes.
$ echo "Hello World"
"Hello World"
$ foo=100
$ echo "I have $foo dollars"
"I have 100 dollars"
$ echo -e "Hellow\\b \\nWorld"
Hello
World
Run in Warp
While echo is straightforward to use, there are noted inconsistencies with its implementation, and is considered “non-portable”. Here is a stackexchange post that elaborates further. Instead, the printf command has become the replacement command and offers a wider range of capabilities in the way it formats and prints the arguments.
printf vs sprintf vs fprintf
In addition to the printf function, there are two other similar functions you may have seen.
printf is arguably the most common and used to print to the standard output stdout
sprintf does not print it to the standard output but rather stores it on a buffer
fprintf does not print to the standard output but rather prints to a file
Written by

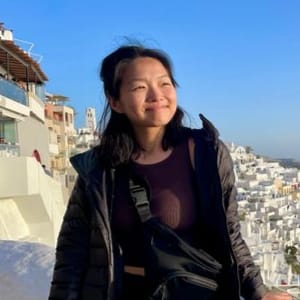
Glory Kim
Software Engineer, Loom
Filed Under
Related Articles
Bash If Statement
Learn how to use the if statement in Bash to compare multiple values and expressions.

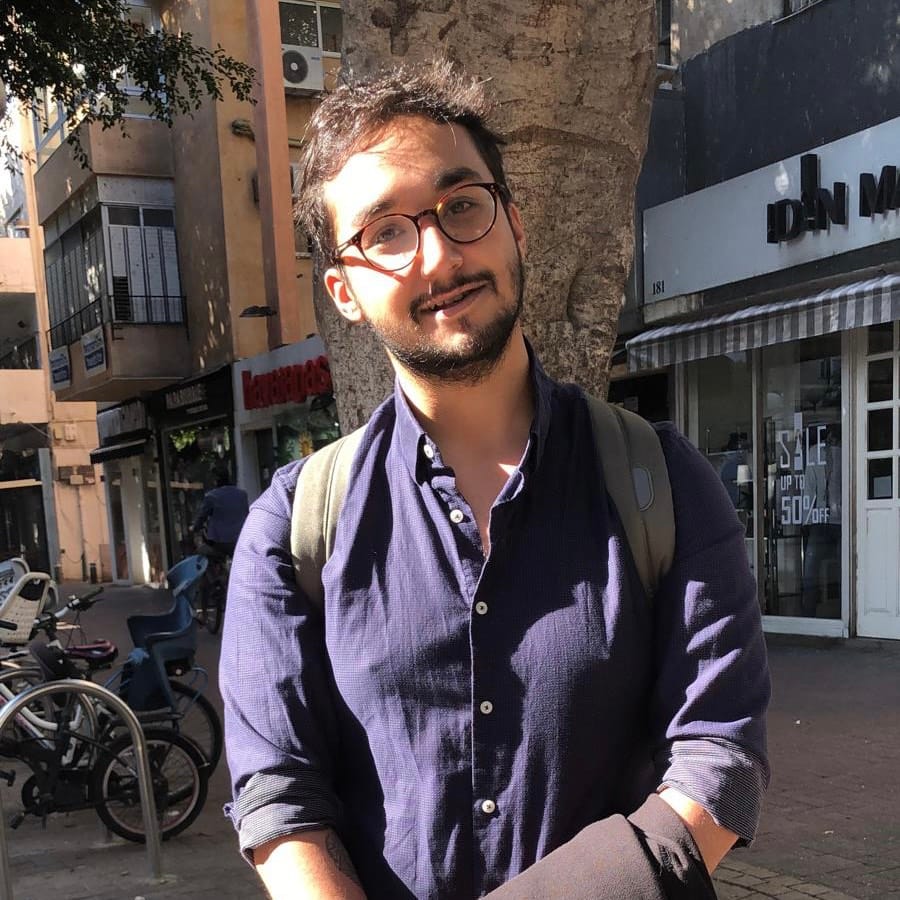
Bash While Loop
Learn how to use and control the while loop in Bash to repeat instructions, and read from the standard input, files, arrays, and more.
POST JSON Data With Curl
How to send valid HTTP POST requests with JSON data payloads using the curl command and how to avoid common syntax pitfalls. Also, how to solve the HTTP 405 error code.

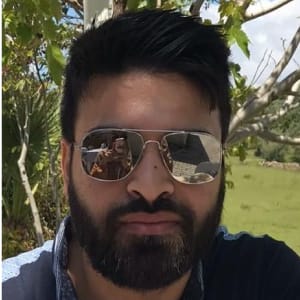
Use Cookies With cURL
Learn how to store and send cookies using files, hard-coded values, environment variables with cURL.
Loop Through Files in Directory in Bash
Learn how to iterate over files in a directory linearly and recursively using Bash and Python.

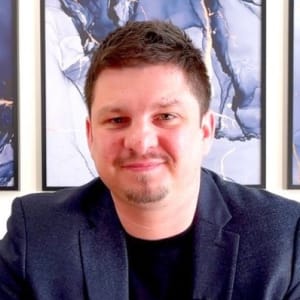
How To Use sudo su
A quick overview of using sudo su

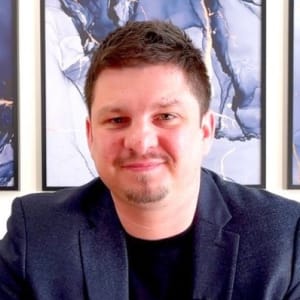
Generate, Sign, and View a CSR With OpenSSL
Learn how to generate, self-sign, and verify certificate signing requests with `openssl`.

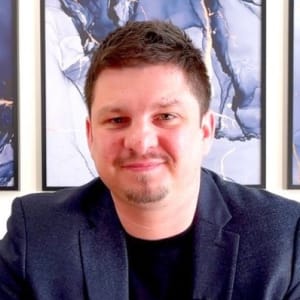
How to use sudo rm -rf safely
We'll help you understand its components

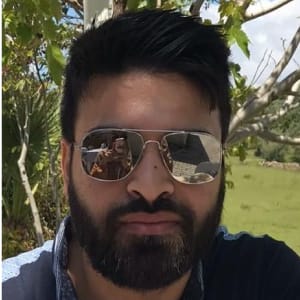
How to run chmod recursively
Using -R is probably not what you want

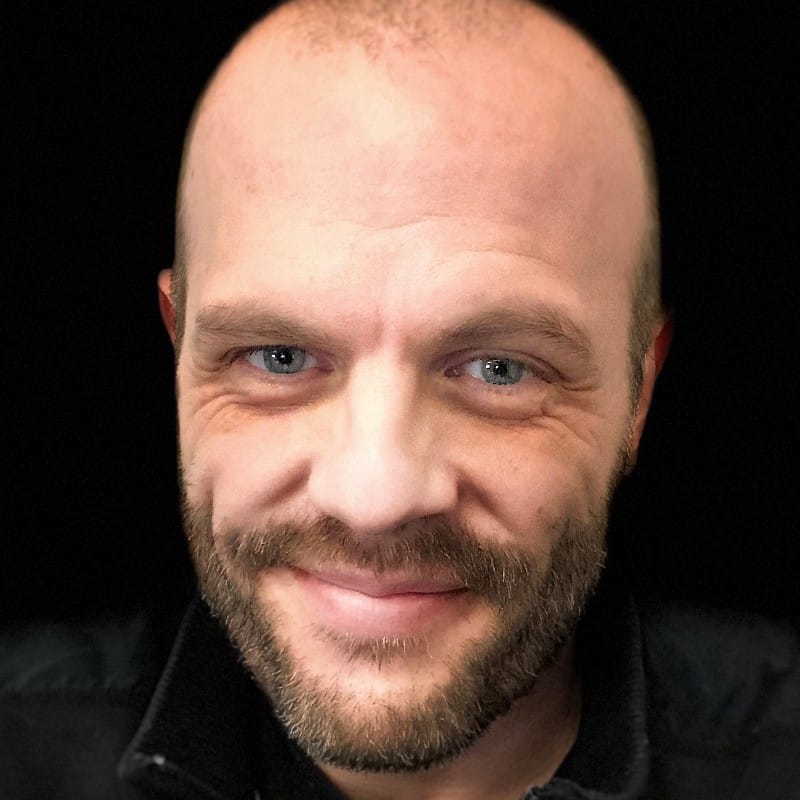
Run Bash Shell In Docker
Start an interactive shell in Docker container

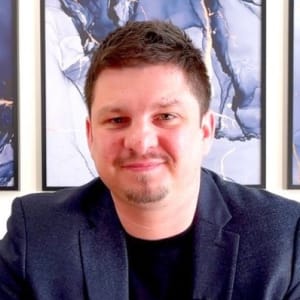
Curl Post Request
Use cURL to send data to a server

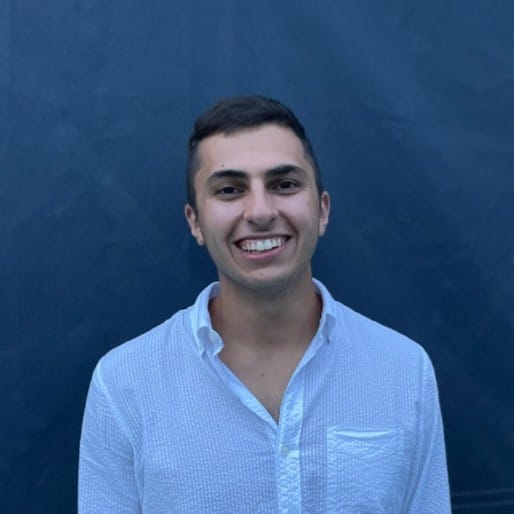
Reading User Input
Via command line arguments and prompting users for input

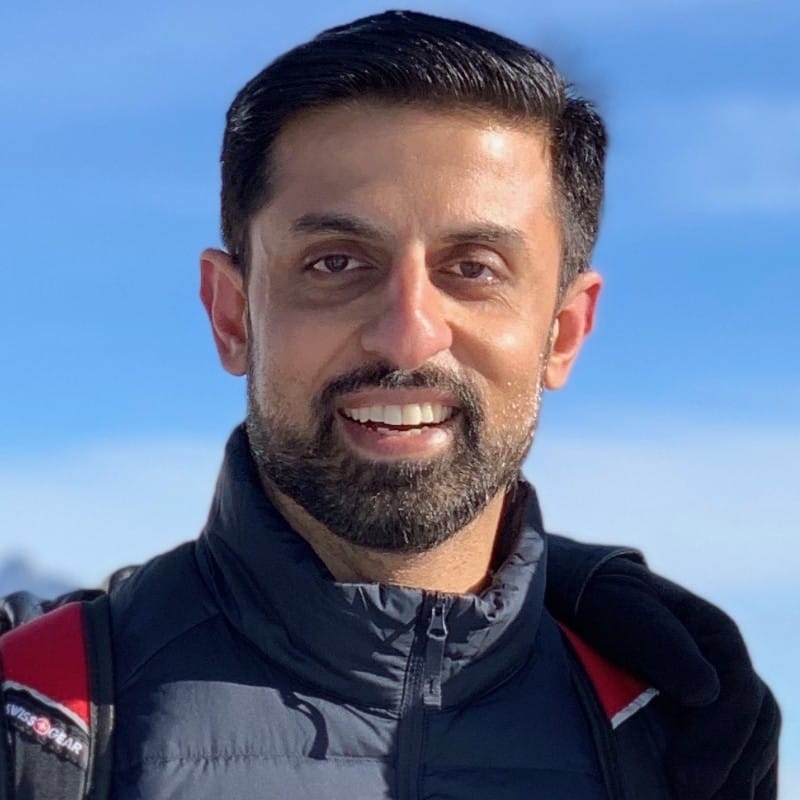