POST JSON Data With Curl
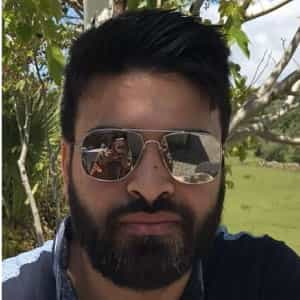
Neeran Gul
Staff Site Reliability Engineer, Mozn
Updated: 7/26/2024
Published: 1/5/2023
The short answer
To send a HTTP request with a payload in the JSON format, you can use the curl command as follows:
$ curl -X POST -H 'Content-Type: application/json' -d '{"<key>":"<value>", ...}' <url>
Where:
- The -X flag is used to set the HTTP method of the request to POST.
- The -H flag is used to set the Content-Type header of the HTTP request to application/json, which corresponds to the JSON media type.
- The -d flag is used to specify the payload of the HTTP request.
For example:
$ curl -X POST -H 'Content-Type: application/json' -d '{"email":"[email protected]","password":"helloworld"}' http://example.com/login
Note that as of curl version 7.82.0, you can also use the --json flag as follows:
$ curl --json '{"<key>":"<value>", ...}' <url>
To learn more about sending HTTP POST requests in general, you can read our other article on how to send POST requests with cURL.
Easily retrieve this command using Warp’s AI Command Suggestions
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestions feature:
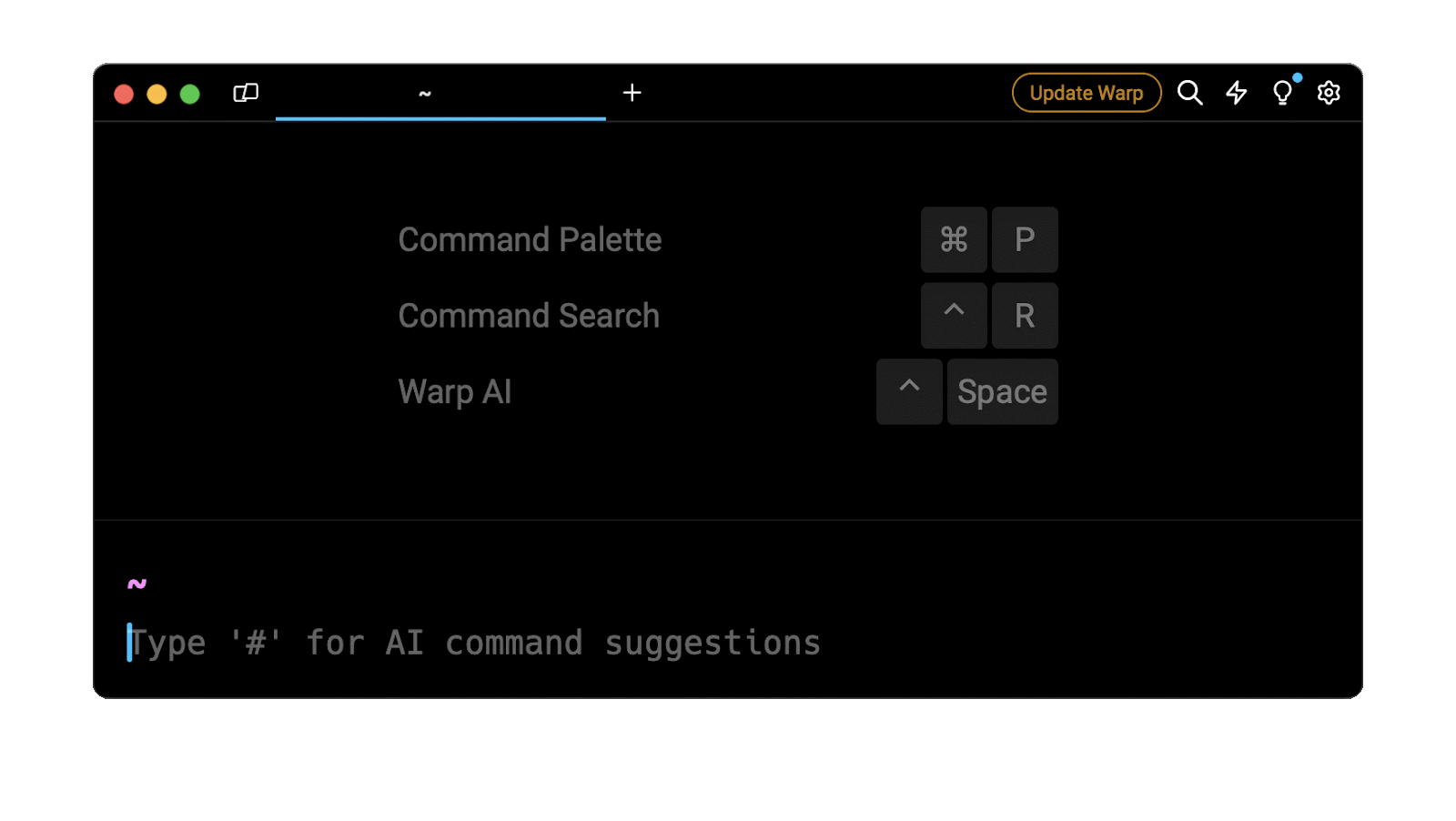
Entering send json data with curl in the AI Command Suggestions will prompt a curl command that can then be quickly inserted in your shell by doing CMD+ENTER .
Sending a JSON payload from a file
To send JSON data from a file instead of the command-line, you can use the following syntax:
$ curl -X POST -H 'Content-Type: application/json' -d @<file> <url>
Where:
- file is the path to the file containing the JSON payload.
For example, this command will send a request with a JSON payload loaded from the file named data.json located in the current working directory:
$ curl -X POST -H 'Content-Type: application/json' -d @data.json http://example.com
Handling large JSON payloads
To send large JSON payloads as it, without any character conversion or modification, you can use the --data-binary flag as follows:
$ curl -X POST -H 'Content-Type: application/json' --data-binary @<file> <url>
Properly formatting the JSON object
Checking the payload format
To make sure that the JSON payload is properly formatted, you can pipe it into the jq command as follows:
$ echo '{"<key>":"<value>", ...}' | jq
$ cat <file> | jq
The jq command will then parse the data, and either write the JSON object back to the terminal if valid, or output an error message otherwise.
For example:
$ echo '{"hello":"world"}' | jq
{
"hello": "world"
}
$ echo '{"hello":world}' | jq
jq: parse error: Invalid numeric literal at line 1, column 15
Escaping special characters in JSON
In JSON, certain characters such as double quotes (") or backslashes () need to be escaped to ensure that the data is correctly formatted and parsed. To escape these characters, you can prepend them with a backslash character.
For example, in the following request, the string h3\o" needs to be escaped as follows:
$ curl -X POST -H 'Content-Type: application/json' -d '{"email":"[email protected]","password":"h3\\o\""}' http://example.com/login
Specifying the payload’s charset
To prevent encoding issues between the client and the server, you can specify the character set of the HTTP request through the Content-Type header as follows:
$ curl -X POST -H 'Content-Type: application/json; charset=UTF-8' -d <payload> <url>
Sending authenticated requests
It may sometimes happen that when sending a request to a protected endpoint, the server responds with an HTTP 401 Unauthorized. This means that the request must be authenticated using an access token, usually generated in response to a login request.
To send an authenticated HTTP request containing an access token, you can specify the Authorization header as follows:
$ curl -X POST \
-H 'Authorization: Bearer <token>' \
-H 'Content-Type: application/json' \
-d '{"<key>":"<value>"}' \
<url>
For example:
$ curl -X POST \
-H 'Authorization: Bearer a8JxldMFxA' \
-H 'Content-Type: application/json' \
-d '{"":""}' \
http://example.com/
Written by
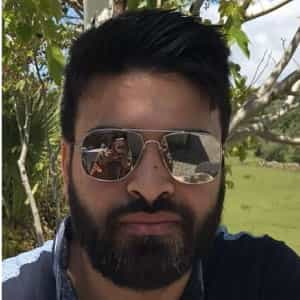
Neeran Gul
Staff Site Reliability Engineer, Mozn
Filed Under
Related Articles
Bash If Statement
Learn how to use the if statement in Bash to compare multiple values and expressions.
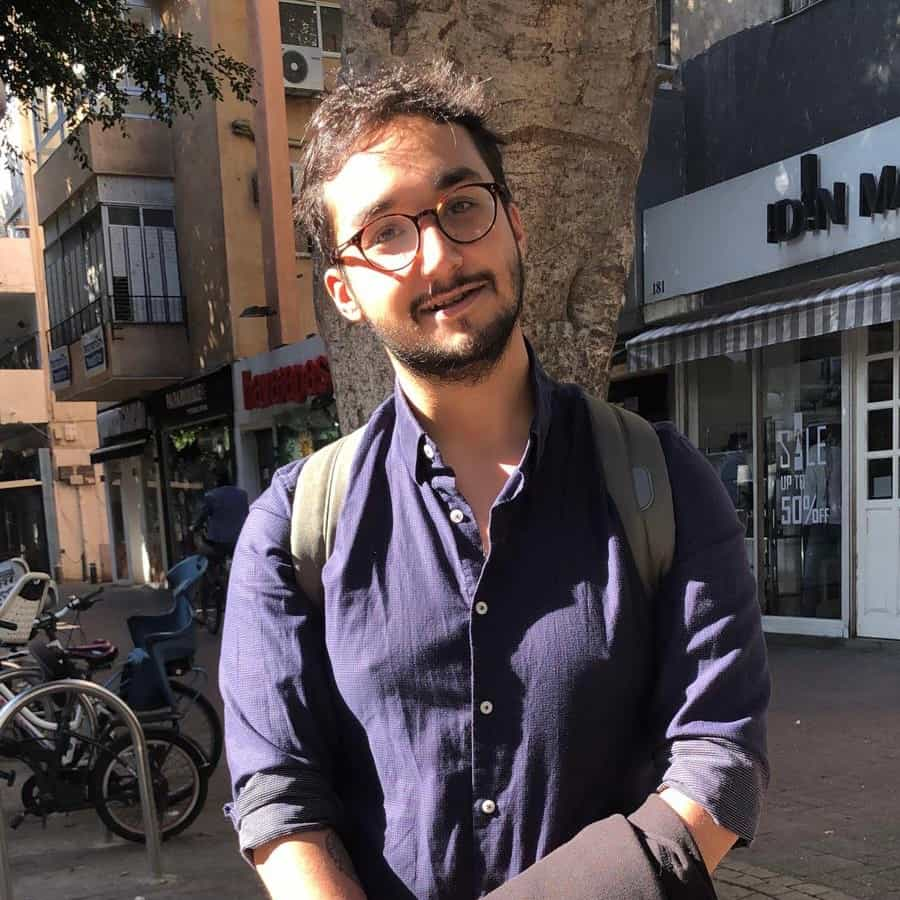
Bash While Loop
Learn how to use and control the while loop in Bash to repeat instructions, and read from the standard input, files, arrays, and more.
Use Cookies With cURL
Learn how to store and send cookies using files, hard-coded values, environment variables with cURL.
Loop Through Files in Directory in Bash
Learn how to iterate over files in a directory linearly and recursively using Bash and Python.
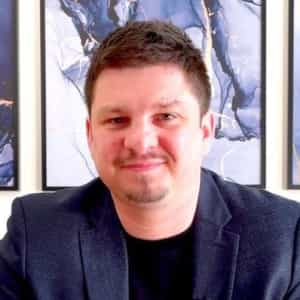
How To Use sudo su
A quick overview of using sudo su
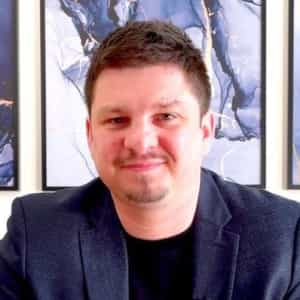
Generate, Sign, and View a CSR With OpenSSL
Learn how to generate, self-sign, and verify certificate signing requests with `openssl`.
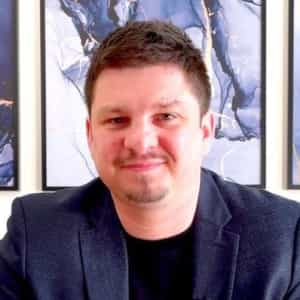
How to use sudo rm -rf safely
We'll help you understand its components
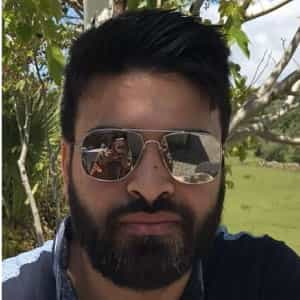
How to run chmod recursively
Using -R is probably not what you want
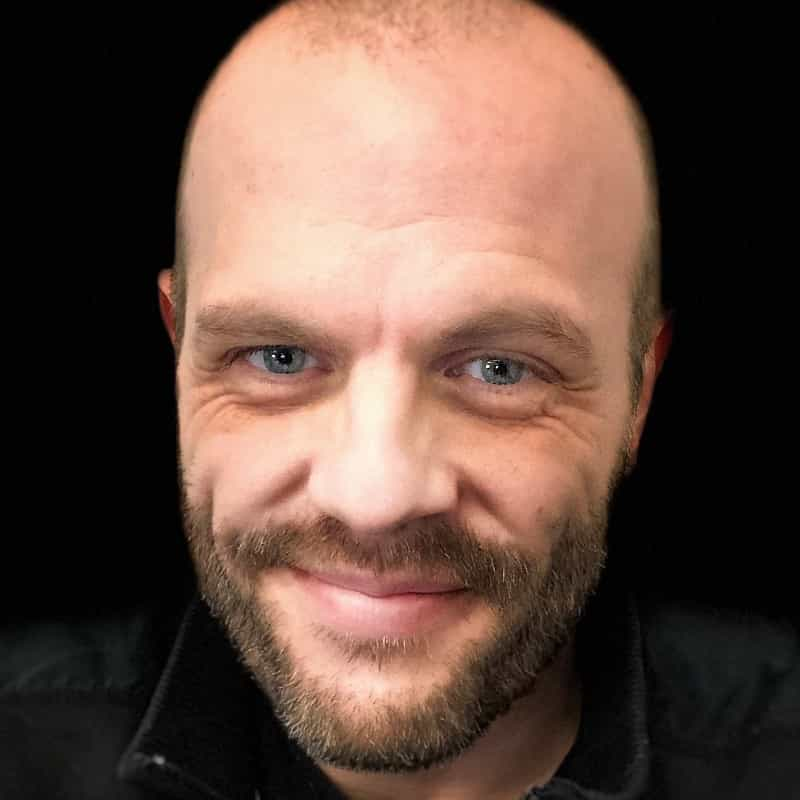
Run Bash Shell In Docker
Start an interactive shell in Docker container
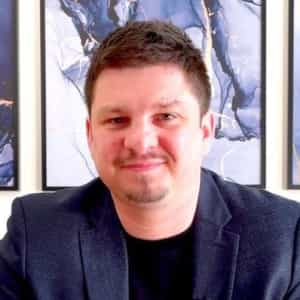
Curl Post Request
Use cURL to send data to a server
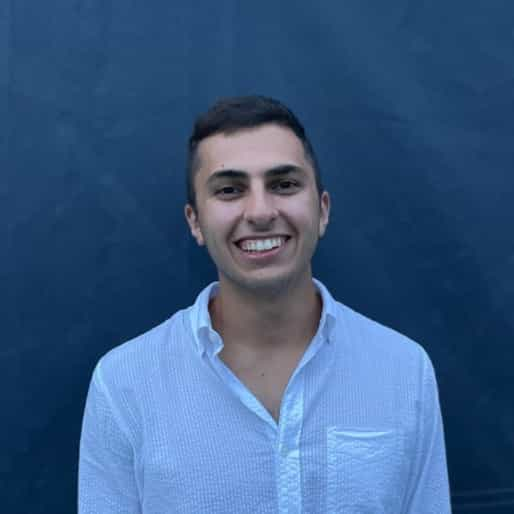
Reading User Input
Via command line arguments and prompting users for input
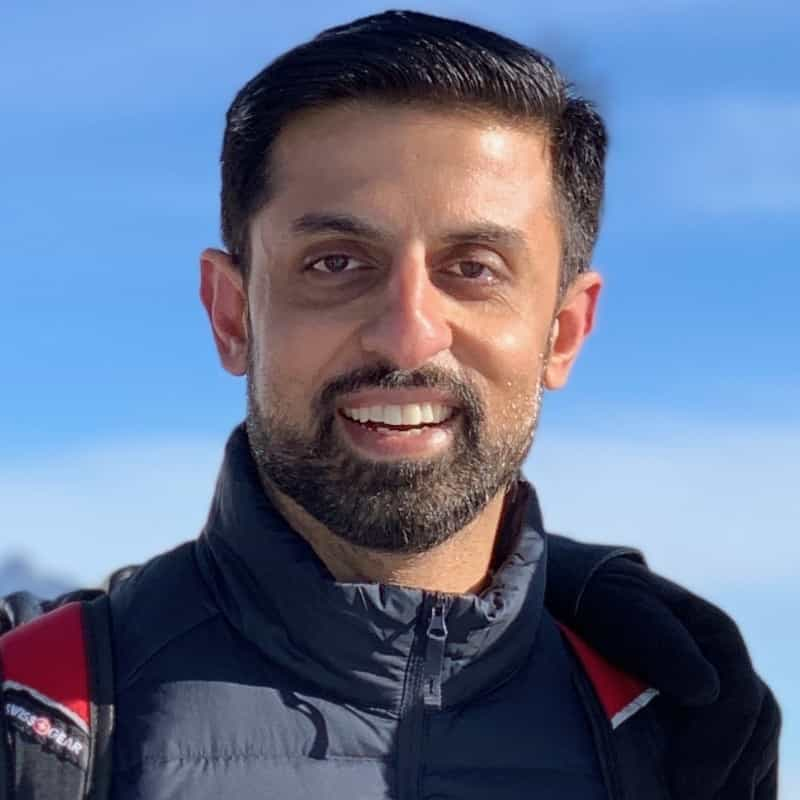
Bash Aliases
Create an alias for common commands
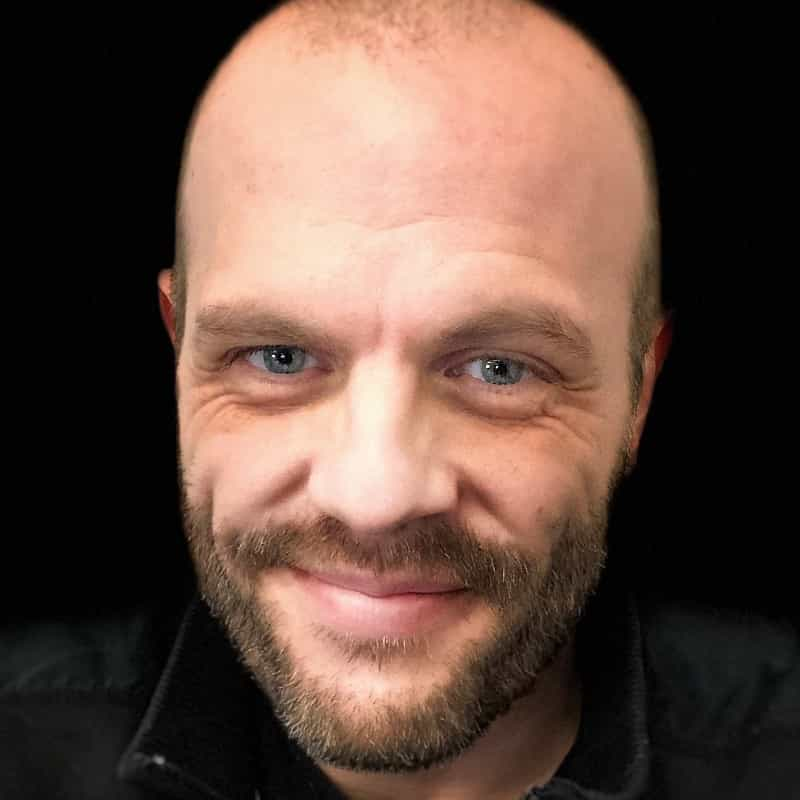