Bash If Statement
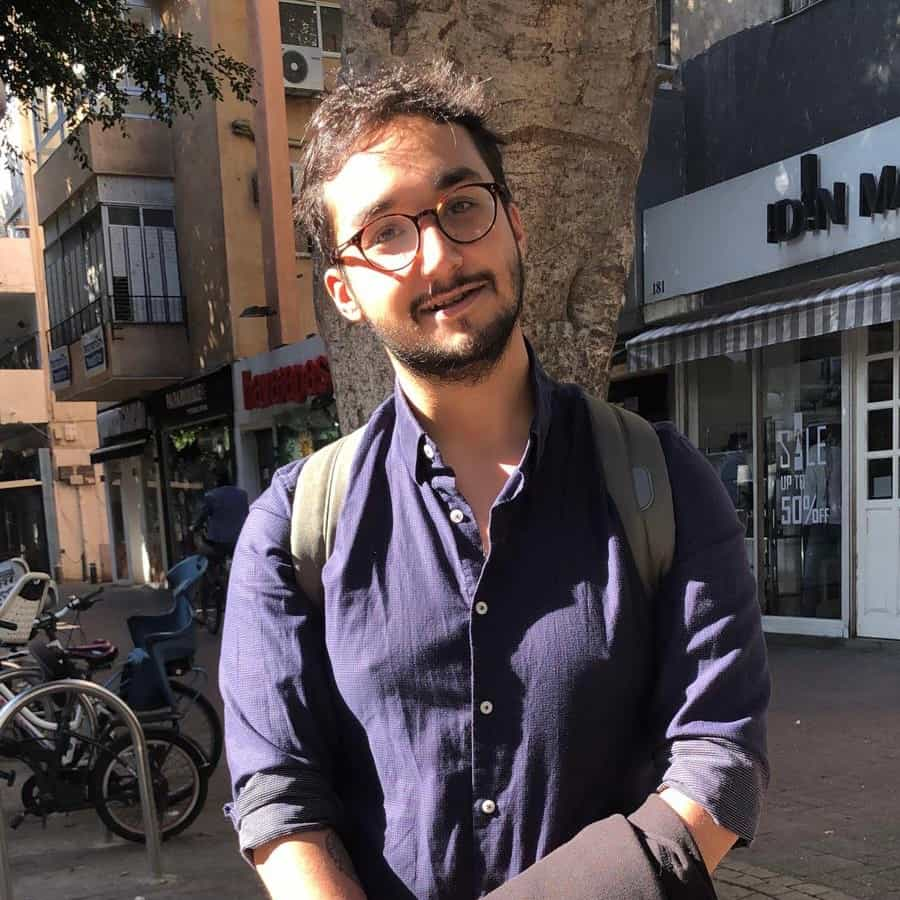
Gabriel Manricks
Chief Architect, ClearX
Updated: 7/30/2024
Published: 7/30/2024
The short answer
In Bash, the if statement is a control structure that allows developers to execute one or more instructions based on the evaluation of a command or an expression called a condition.
If the condition evaluates to true (i.e. the exit status equals zero), then the subsequent instructions are executed.
The syntax of the if statement is as follows:
if condition; then
instructions
fi
Where:
- if starts the conditional statement.
- condition is the expression that will be evaluated.
- then specifies the set of instructions to execute if the condition is true.
- fi ends the conditional statement.
For example, let's consider the following script, where the echo command will only be executed if the exit status of the grep command is 0 :
#!/bin/bash
if grep -q error access.log; then
echo "log contains errors"
fi
Comparing values
In order to compare two values together within an if statement, you need to enclose the comparison expression within square brackets [ as follows:
if [ expression ]; then
instructions
fi
Where:
- expression is a logical expression composed of variables, values, and comparison operators.
Note that this syntax is in fact an alias for the built-in test command.
Comparing numbers
Numeric comparisons are done using the following operators:
- -eq : equal to
- -ne : not equal to
- -lt : less than
- -le : less than or equal to
- -gt : greater than
- -ge : greater than or equal to
For example, let's consider this script that makes the user guess a number:
#!/bin/bash
number=5
while true;
do
read -p "Guess the number: " input
if [ $input -lt $number ]; then
echo "Too low..."
continue
fi
if [ $input -gt $number ]; then
echo "Too high..."
continue
fi
if [ $input -eq $number ]; then
echo "Good guess!"
break
fi
done
Comparing strings
String comparisons are done using the following operators:
- = : equal to
- != : not equal to
- < : less than
-
: greater than
And the following flags:
- -z : string is empty
- -n : string is not empty
Note that when using flags, you can use the NOT ! operator to negate the expression.
For example, let’s consider this script that makes the user guess a word:
#!/bin/bash
word="hello"
echo "Guess the word (type 'quit' to exit)."
while :
do
read -p "> " input
if [ ! -n $input ]; then
echo "Error: invalid input"
continue
fi
if [ $input == "quit" ]; then
echo "Goodbye!"
break
fi
if [ $input != $word ]; then
echo "Try again..."
continue
fi
if [ $input == $word ]; then
echo "Congratulations!"
break
fi
done
Combining conditions
The logical && (AND) and || (OR) operators allow you to combine two or more conditions into a single expression.
The logical AND
When using the AND operator, all conditions must evaluate to true for the subsequent instructions to be executed:
if [ condition ] && [ condition ]; then
# instructions
fi
The logical OR
When using the OR operator, only one of the conditions must evaluate to true for the subsequent instructions to be executed:
if [ condition ] || [ condition ]; then
# instructions
fi
Evaluating complex conditions
Unlike the single square brackets [ , the double square brackets [[ provide a more advanced processing and evaluation of conditional expressions.
It retrieves the specified expression as a single string instead of separate arguments, and performs its own expansions.
if [[ expression ]]; then
# instructions
fi
Matching simple and regex patterns
In addition, the double square brackets allow you to match simple and advanced patterns using the =~ operator.
Simple matchers include:
- [<chars>] : a specific set of characters
- ? : a single character
- * : any string/substring
Note that these matchers need to be placed outside of quotes as they will otherwise lose their meaning and be treated as regular characters.
For example, let’s consider this script that checks whether the file passed as argument of the script is a JavaScript or JSON file:
#!/bin/bash
if [[ "$1" =~ \.(js|json)$ ]]; then
echo "Valid JS or JSON file"
fi
Evaluating arithmetic expressions
To easily perform basic arithmetic operations within an if statement using mathematical operators, such as + , - , * , etc, and comparison operators, such as > , <= , == , etc, you can use the double parentheses (( instead of the square brackets [[ as follows:
if (( expression )); then
# instructions
fi
For example, [text]:
#!/bin/bash
number=1
i=2
if (( $number <= 2 )); then
echo "not prime"
exit 1
fi
while [ $i -lt $number ];
do
if (( $number % $i == 0 )); then
echo "not prime"
exit 1
fi
((i++))
done
echo "prime"
Testing files and directories
Testing the existence
To test the existence and type of files or directories you can respectively use the -f and -d flags.
For example:
if [ -f ./script.sh ]; then
# instructions
fi
if [ -d /home/johndoe ]; then
# instructions
fi
Testing permissions
To test whether a file or directory has read, write, or execute permission, you can respectively use the -r , -w , and -x flags.
if [[ ! -x ./my_script.sh ]]; then
chmod +x my_script.sh
fi
Written by
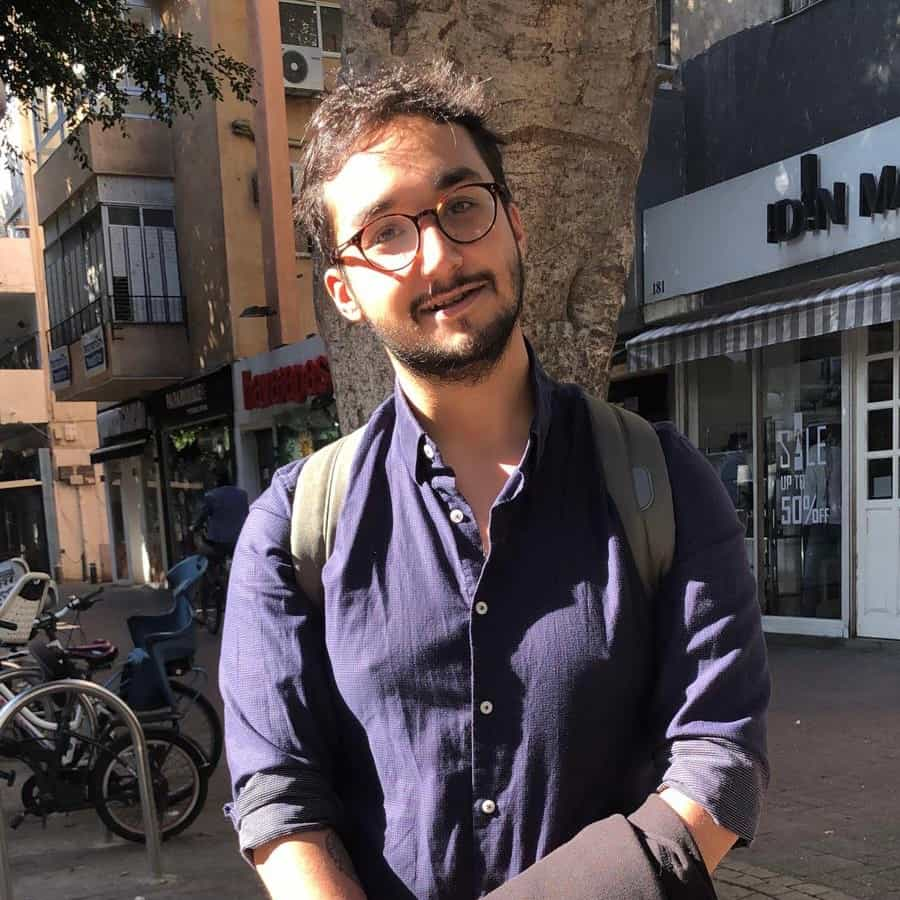
Gabriel Manricks
Chief Architect, ClearX
Filed Under
Related Articles
Bash While Loop
Learn how to use and control the while loop in Bash to repeat instructions, and read from the standard input, files, arrays, and more.
POST JSON Data With Curl
How to send valid HTTP POST requests with JSON data payloads using the curl command and how to avoid common syntax pitfalls. Also, how to solve the HTTP 405 error code.
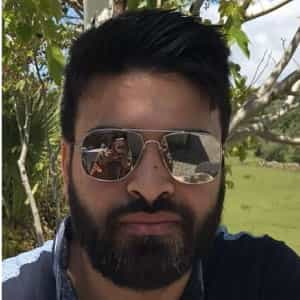
Use Cookies With cURL
Learn how to store and send cookies using files, hard-coded values, environment variables with cURL.
Loop Through Files in Directory in Bash
Learn how to iterate over files in a directory linearly and recursively using Bash and Python.
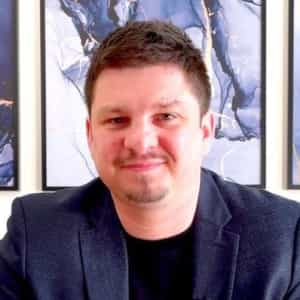
How To Use sudo su
A quick overview of using sudo su
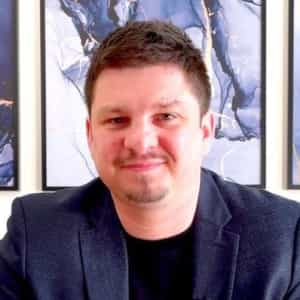
Generate, Sign, and View a CSR With OpenSSL
Learn how to generate, self-sign, and verify certificate signing requests with `openssl`.
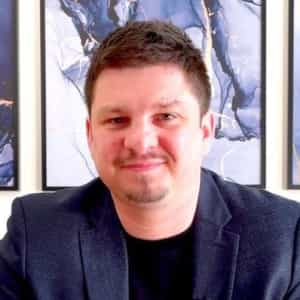
How to use sudo rm -rf safely
We'll help you understand its components
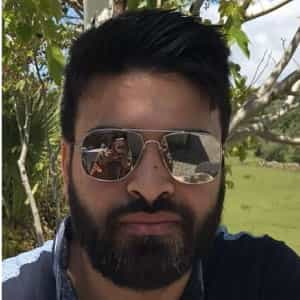
How to run chmod recursively
Using -R is probably not what you want
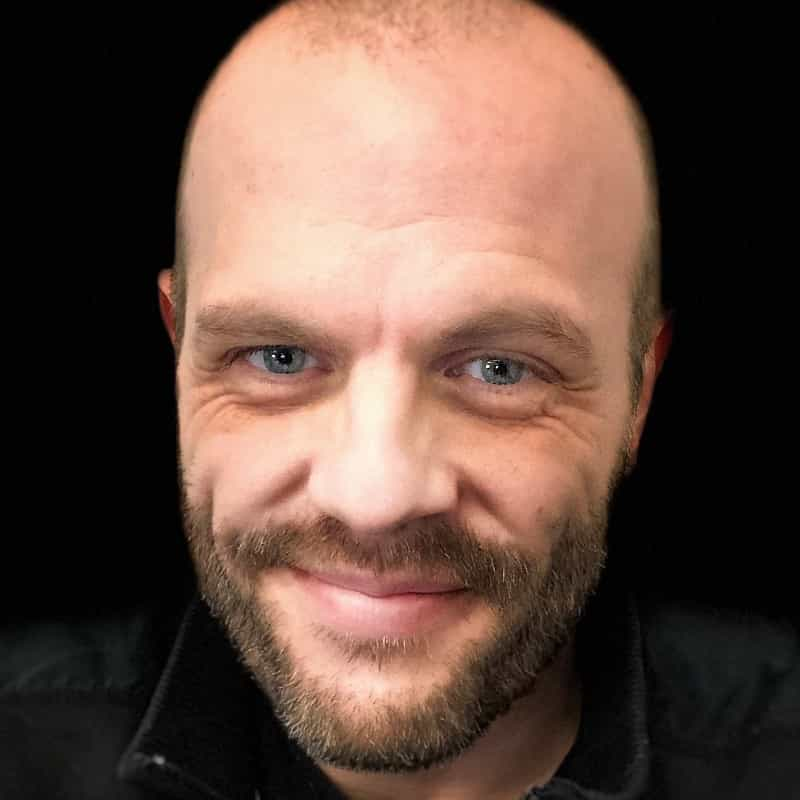
Run Bash Shell In Docker
Start an interactive shell in Docker container
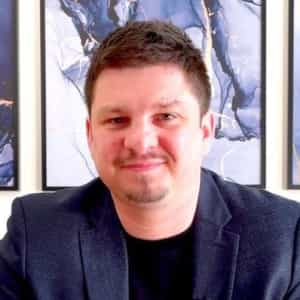
Curl Post Request
Use cURL to send data to a server
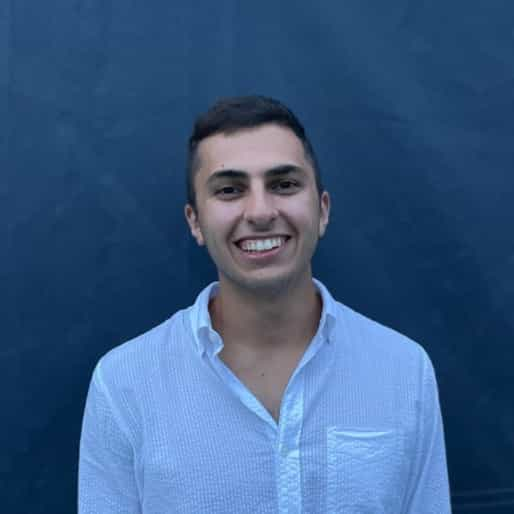
Reading User Input
Via command line arguments and prompting users for input
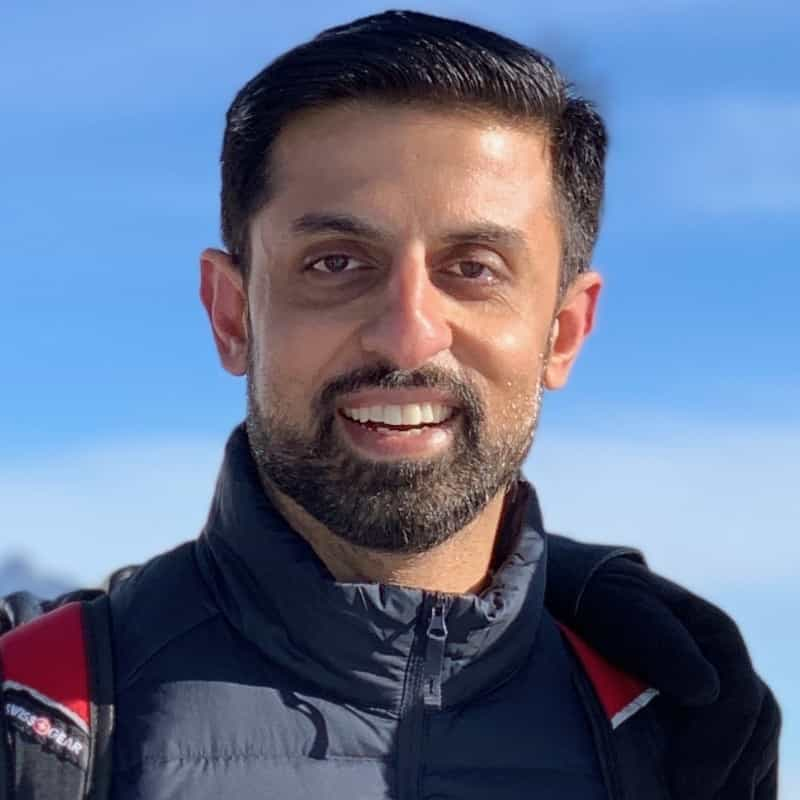
Bash Aliases
Create an alias for common commands
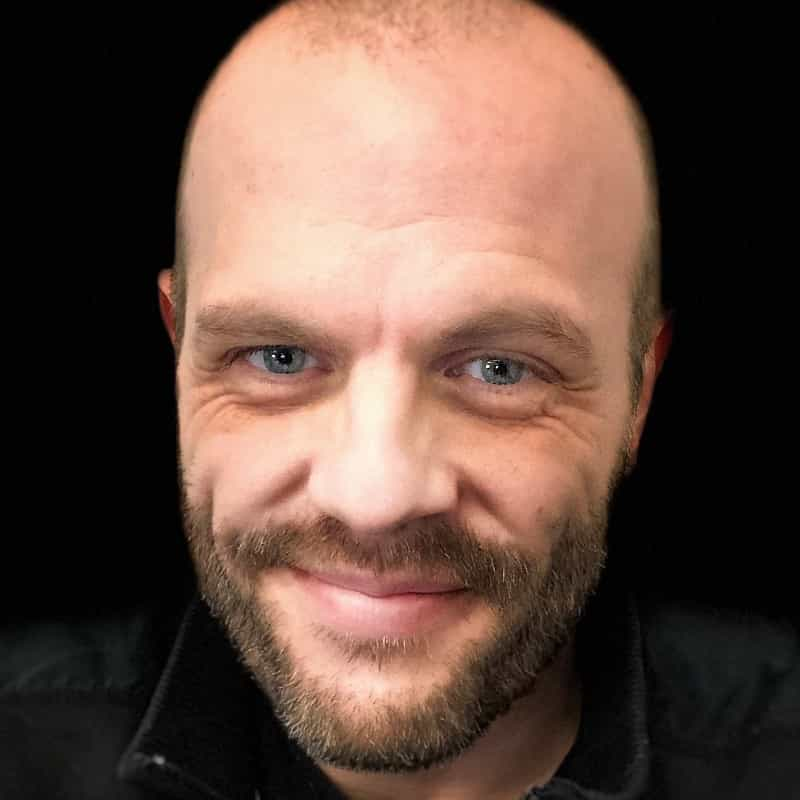