The short answer
On Unix-like operating systems such as macOS and Linux, you can create one or more empty files using the [.inline-code]touch[.inline-code] command as follows:
Where:
- [.inline-code]file ...[.inline-code] is a list of paths to the file you want to create.
For example, this command will create a new empty file named [.inline-code]test_script.sh[.inline-code] in the current working directory:
And this command will create two new empty files named [.inline-code]index.js[.inline-code] and [.inline-code]package.json[.inline-code] in the [.inline-code]website[.inline-code] directory located in the home directory:
Note that if the destination file already exists, only the file’s access and modification time will be updated.
Should you encounter “permission denied” errors while trying to create a file, check that you have the necessary permissions to create files in the target folder.
You can learn more about this in our other articles, Linux File Permissions Explained and Linux Chmod Command.
[#create-a-file-with-content] Writing content in a new file using the output redirection operator [#create-a-file-with-content]
In Unix-like operating systems, the output redirection operator ([.inline-code]>[.inline-code]) is used to redirect the output of a command so that it writes into a file instead of the standard output of the terminal.
[#write-a-string-in-a-file-with-echo] Writing a string in a new file using [.inline-code]echo[.inline-code] [#write-a-string-in-a-file-with-echo]
To write an arbitrary string into a new file, you can use the [.inline-code]echo[.inline-code] command combined with the output redirection operator as follows:
Where:
- [.inline-code]string[.inline-code] is a string of characters.
- [.inline-code]>[.inline-code] is the output redirection operator.
- [.inline-code]file[.inline-code] is the path to the file you want the [.inline-code]echo[.inline-code] command to write the [.inline-code]string[.inline-code] into.
For example, this command will write the string "NODE_ENV=development” into the file named [.inline-code].env.development[.inline-code] located in the current working directory:
Note that if the destination file already exists, the output redirection operator will overwrite its content with the specified string.
You can learn more about appending content to an existing file by reading our other article on how to edit files in Linux.
[#easily-recall-the-syntax-with-ai] Easily retrieve this command using Warp’s AI Command Suggestions [#easily-recall-the-syntax-with-ai]
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestions feature:
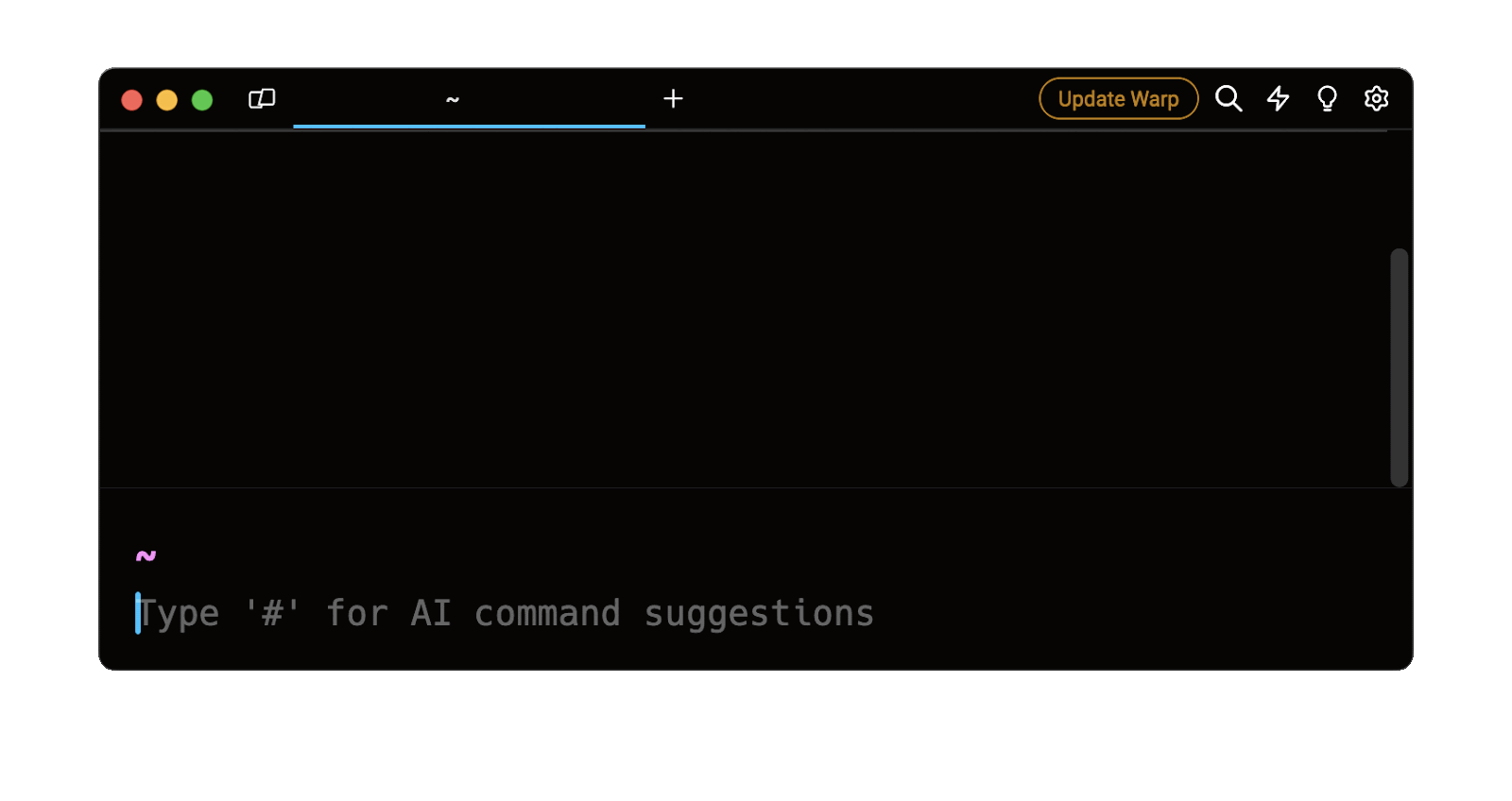
Entering [.inline-code]write strings into a file[.inline-code] in the AI Command Suggestions will prompt an [.inline-code]echo[.inline-code] command that can then quickly be inserted into your shell by doing [.inline-code]CMD+ENTER[.inline-code].
[#write-multiple-strings-in-a-file-with-cat] Writing multiple strings in a new file using [.inline-code]cat[.inline-code] [#write-multiple-strings-in-a-file-with-cat]
Although the [.inline-code]cat[.inline-code] command is typically used to display the content of files, you can also use it to create text files interactively using the following syntax:
Where:
- [.inline-code]file[.inline-code] is the path to the file you want the [.inline-code]cat[.inline-code] command to write into.
When executed, the [.inline-code]cat[.inline-code] command will read from the standard input until it encounters an EOF (end-of-file) sequence, which can be triggered by pressing the [.inline-code]CTRL + D[.inline-code] key combination:
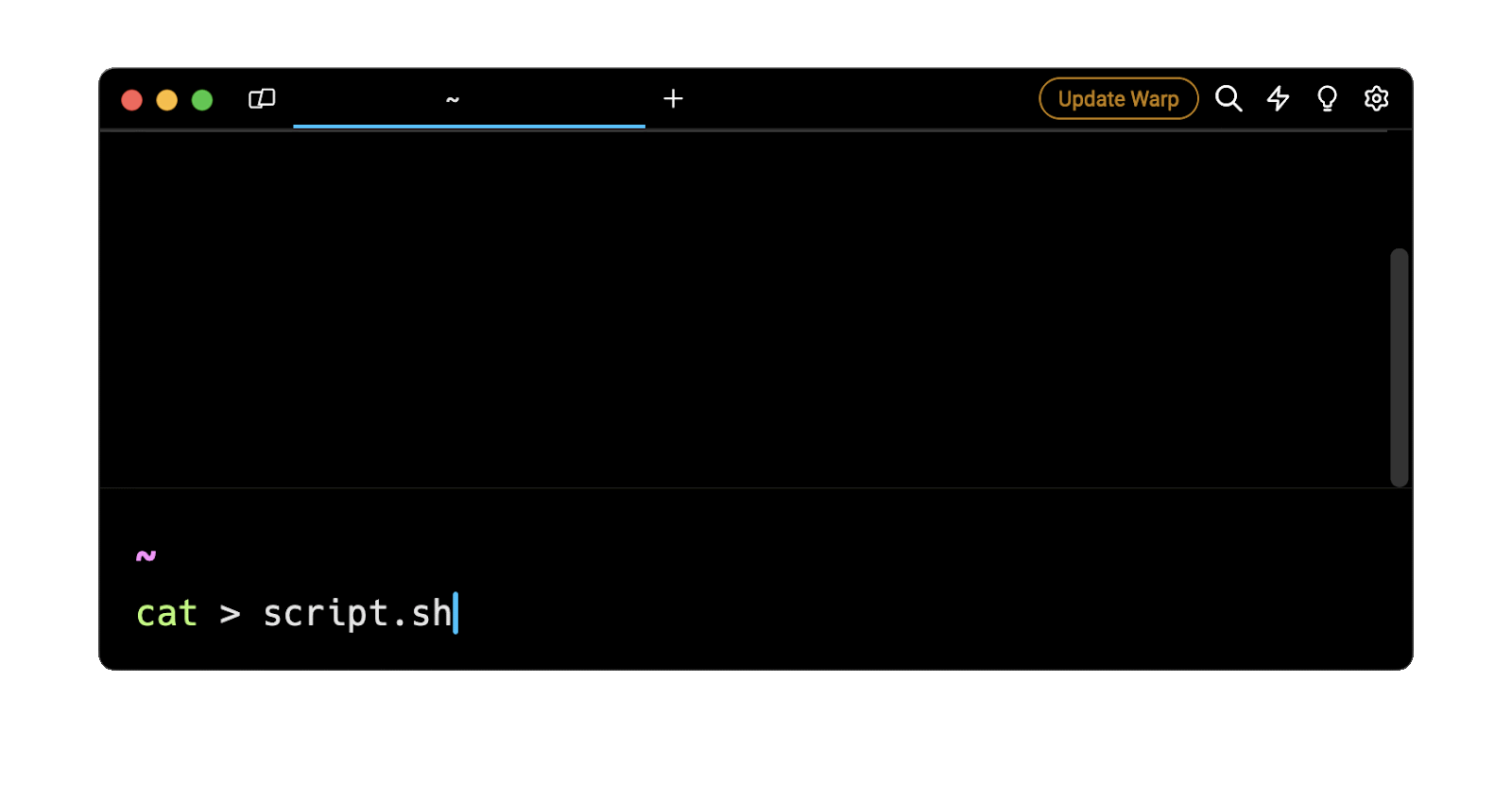
Note that when using the [.inline-code]cat[.inline-code] command, the newlines will automatically be preserved.
[#write-a-formatted-string-in-a-file-with-printf] Writing a formatted string in a new file using [.inline-code]printf[.inline-code] [#write-a-formatted-string-in-a-file-with-printf]
To write a formatted string into a new file, you can use the [.inline-code]printf[.inline-code] command as follows:
Where:
- [.inline-code]format[.inline-code] is a format string that includes characters and specifiers such as [.inline-code]%s[.inline-code] to specify a string of characters, [.inline-code]%d[.inline-code] to specify an integer, [.inline-code]%f[.inline-code] to specify a float, and so on.
- [.inline-code]value ...[.inline-code] represents a list of values that will replace the specifier in the [.inline-code]format[.inline-code] string, in the order of declaration.
For example, this command will create a new file named [.inline-code]project_info.txt[.inline-code] in the current working directory:
With the following content:
[#create-a-file-with-vim] Creating a new file using Vim [#create-a-file-with-vim]
When you need to work with larger and more complex texts, you can use in-terminal Vim text editor, which is by default installed on most Linux distributions.
To create a new file with Vim, you can use the [.inline-code]vim[.inline-code] command as follows:
Where:
- [.inline-code]file[.inline-code] is the path to the file you want to create.
Once launched, you can:
- Press the [.inline-code]i[.inline-code] key to enter the [.inline-code]INSERT[.inline-code] mode.
- Type your text, code, etc.
- Press the [.inline-code]ESCAPE[.inline-code] key to come back to the default [.inline-code]NORMAL[.inline-code] mode.
- Type [.inline-code]:wq[.inline-code] command to save your changes and quit the editor.
For example:
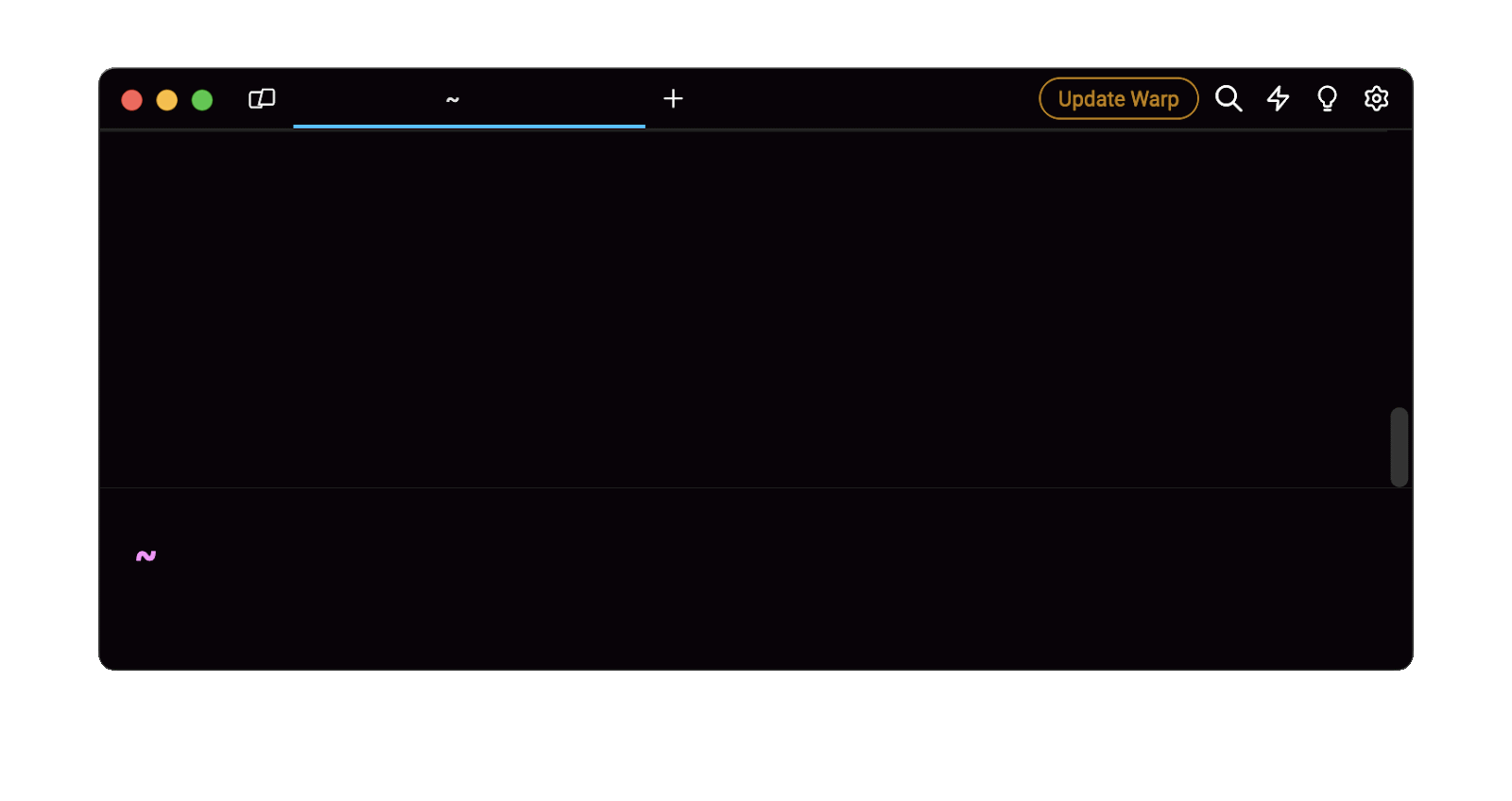
You can learn more about how Vim works by reading our other articles on Vim modes and how to edit files in Linux.
[#create-a-file-with-spaces-in-the-filename] Creating a new file with spaces in the filename [#create-a-file-with-spaces-in-the-filename]
To create a new file with space characters in the filename, you can either escape the spaces using a backslash character ([.inline-code]\[.inline-code]) as follows:
Or enclose the filename in single or double quotes as follows:
Note that including space characters in filenames is usually not recommended as it usually makes it harder to work with files.
[#create-a-file-of-a-predefined-size] Creating a new file with a predefined size [#create-a-file-of-a-predefined-size]
To create a new file of a predefined size, rather than filling it will zeros, you can use the [.inline-code]fallocate[.inline-code] command with the [.inline-code]-l[.inline-code] flag (short for [.inline-code]--length[.inline-code]) as follows:
Where:
- [.inline-code]size[.inline-code] is the size of the file suffixed with a letter such as [.inline-code]K[.inline-code] for kilobytes, [.inline-code]M[.inline-code] for megabytes, [.inline-code]G[.inline-code] for gigabytes, and so on.
- [.inline-code]file[.inline-code] is the path to the file you want to create.
For example, this command will create a new empty file named [.inline-code]data.txt[.inline-code] in the current working directory with a size of [.inline-code]500[.inline-code] kilobytes:
[#create-a-file-with-a-bash-script] Automating the creation of new files using a Bash script [#create-a-file-with-a-bash-script]
To automate the creation of a new file and prevent the overwriting of existing ones, you can use a Bash script as follows:
Where:
- [.inline-code]#!/bin/bash[.inline-code] is a directive used to indicate that the script must be executed using the Bash binary.
- [.inline-code]FILEPATH="path/to/file"[.inline-code] is a variable used to store the path to the file you want to create.
- [.inline-code][[ ! -f $FILEPATH ]][.inline-code] is a condition used to check if the file already exists.
Note that to execute this script, you must first give it execution permission using the [.inline-code]chmod[.inline-code] command:
Then execute it using the following command: