How To Launch A PostgreSQL Container In Docker
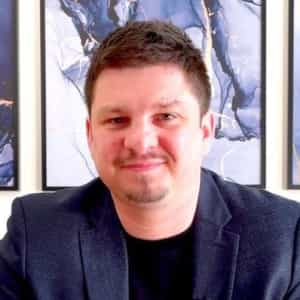
Razvan Ludosanu
Founder, learnbackend.dev
Published: 1/31/2024
The short answer
In Docker, to launch a PostgreSQL container, you can use the following docker run command:
$ docker run -d \
-e POSTGRES_PASSWORD=<password> \
-p <host_port>:<container_port>
postgres
Where:
- The -d flag is used to run the container in detached mode (i.e. in the background).
- The -e flag is used to define environment variables for the container.
- The POSTGRES_PASSWORD variable is used to set the PostgreSQL root password.
- The -p flag is used to map the ports of the host and the container.
- The host_port variable is the port on the host machine you want to map.
- The container_port variable is the port on the container you want to expose.
For example:
$ docker run -d -e POSTGRES_PASSWORD=admin -p 5432:5432 postgres
This command launches the latest version of PostgreSQL in a Docker container in detached mode that matches the port 5432 of the host to the port 5432 of the container and sets the root username to postgres (default) and the root password to admin.
If you are working with multi-container applications, you can read our article on how to launch PostgreSQL with Docker Compose.
Easily retrieve this command using Warp’s AI Command Suggestions
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestions feature:
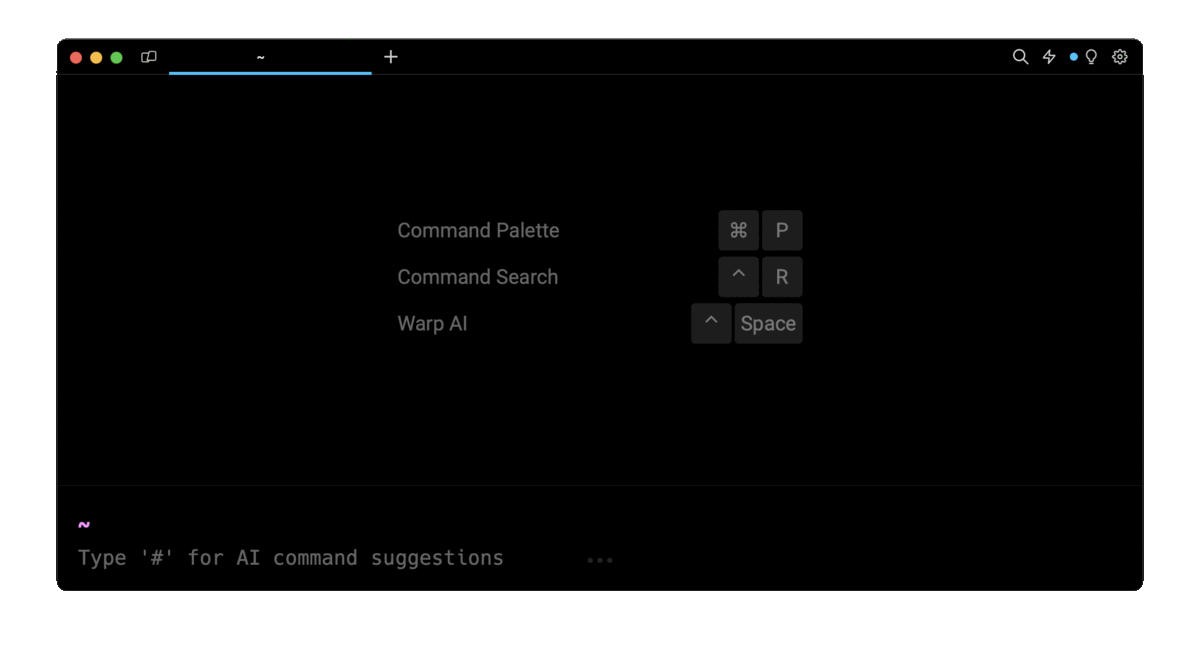
Entering docker run postgres in the AI Command Suggestions will prompt a docker command that can then quickly be inserted into your shell by doing CMD+ENTER.
Connecting to a Postgres container
To connect to a PostgreSQL instance running within a Docker container, you can use the docker exec command combined with the psql command:
$ docker exec -it <container> psql -U <username>
Where:
- container is the name or identifier of the Postgres container retrieved using the docker ps command.
- username is the name of the Postgres user you want to connect to the database with.
Once connected, you can then use commands such as \l to list the databases or \dt to list the tables within the selected database.
For example:
$ docker exec -it database psql -U postgres
This command executes the psql -U command within the Postgres container named database using the default Postgres user named postgres.
Creating a Postgres user
The default user created by the postgres image when launching a container is named postgres.
To create a specific user with superuser privileges, you can use the POSTGRES_USER environment variable as follows:
$ docker run -d \
-e POSTGRES_USER=<username> \
-e POSTGRES_PASSWORD=<password> \
-p <host_port>:<container_port> \
postgres
Note that, the password defined in the POSTGRES_PASSWORD variable will be assigned to the user defined in the POSTGRES_USER variable.
For example:
$ docker run -d \
-e POSTGRES_USER=admin \
-e POSTGRES_PASSWORD=admin \
-p 5432:5432 \
postgres
This command launches a Postgres container with a new root user named admin, identified by the password admin.
Creating a custom database
By default, Postgres creates a database matching the name of the user defined in the POSTGRES_USER variable.
To define a different name for the default database that is created when the image is first started, you can use the POSTGRES_DB variable as follows:
$ docker run -d\
-e POSTGRES_USER=<username>\
-e POSTGRES_PASSWORD=<password>\
-e POSTGRES_DB=<database>\
-p <host_port>:<container_port>\
postgres
For example:
$ docker run -d \
-e POSTGRES_USER=admin \
-e POSTGRES_PASSWORD=admin \
-e POSTGRES_DB=myproject \
-p 5432:5432 postgres
This command launches a Postgres container with a new root user named admin, identified by the password admin, whose default database is myproject.
Persisting the database data
By default, all the data present in a container's file system is automatically discarded as soon as the container is stopped and removed.
To preserve the database data on your local machine, you can either create a named volume, which is a data storage mechanism entirely managed by Docker, or a bind mount, which allows you to tie together a file on the host to a file within the container.
Using a named volume
To persist the Postgres data in a named volume, you can launch the Postgres container using the docker run command with the -v flag (short for --volume) as follows:
$ docker run -d \
-e POSTGRES_PASSWORD=<password> \
-v <volume>:/var/lib/postgresql/data \
-p <host_port>:<container_port> \
postgres
Where:
- volume is the name of the volume.
For example:
$ docker run -d \
-e POSTGRES_PASSWORD=admin \
-v pgdata:/var/lib/postgresql/data \
-p 5432:5432 \
postgres
This command launches a Postgres container that stores the data generated by the container in the /var/lib/postgresql/data directory into the pgdata volume on the host.
Note that when using this command, Docker will automatically create the specified named volume if it doesn't exist.
Using a bind mount
On the other hand, to persist the Postgres data using a bind-mounted directory, you can launch the Postgres container using the docker run command with the -v flag (short for --volume) as follows:
$ docker run -d \
-e POSTGRES_PASSWORD=<password> \
-e PGDATA=/var/lib/postgresql/data/pgdata \
-v <host_mount_path>:/var/lib/postgresql/data \
-p <host_port>:<container_port> \
postgres
Where:
-
PGDATA is an environment variable used to specify the path to the subdirectory containing the database files within the container.
-
host_mount_path is the path to the bind-mounted directory on the host.
Note that, if the data volume you're using is a filesystem mountpoint, Postgres requires a subdirectory to be created within that mountpoint to contain the data.
For example:
$ docker run -d \
-e POSTGRES_PASSWORD=admin \
-e PGDATA=/var/lib/postgresql/data/pgdata \
-v /tmp/data:/var/lib/postgresql/data \
-p 5432:5432 \
postgres
This command launches a Postgres container that stores the data generated by the container in the /var/lib/postgresql/data directory into the /tmp/data directory on the host.
You can learn more about persisting data in Docker with our article on how to create bind mounts.
Connecting pgAdmin to Postgres
To manage your Postgres database using the pgAdmin interface, you can start by launching a pgAdmin container using the following docker run command:
$ docker run -d \
-e PGADMIN_DEFAULT_EMAIL=<email> \
-e PGADMIN_DEFAULT_PASSWORD=<password> \
-p <host_port>:<container_port> \
dpage/pgadmin4
Where:
- PGADMIN_DEFAULT_EMAIL is used to create a new user account on pgAdmin.
- PGADMIN_DEFAULT_PASSWORD is used as a password for the user account identified by PGADMIN_DEFAULT_EMAIL.
Then connect to the pgAdmin interface by navigating to the following address 127.0.0.1:<host_port> in your web browser.
For example:
$ docker run -d \
-e [email protected] \
-e PGADMIN_DEFAULT_PASSWORD=mysecret \
-p 8080:80
dpage/pgadmin4
This command launches a pgAdmin container in detached mode based on the dpage/pgadmin4 image, that maps the port 8080 of the host to the port 80 (i.e. HTTP) of the container, to which you can connect to using the [email protected] email and mysecret password.
Running a SQL script
To run an SQL script against a containerized Postgres application, you can start by copying the script into the container using the docker cp command:
$ docker cp <src_path> <container>:<dst_path>
Where:
- src_path is the path on your local machine of the SQL script you want to copy.
- container is the name or the ID of the container you want to copy files to.
- dest_path is the path in the container of the directory you want to copy the script to.
Then, connect into the container using the docker exec command:
$ docker exec -it <container> /bin/bash
Where:
- container is the name or the ID of the container you want to connect into.
And finally, import the script into the Postgres database using the psql command with the input redirection operator (<):
$ psql -U <username> -d <database> < <script>
Where:
- username is the name of the user you want to connect to the database as.
- database is the database you want to execute the script against.
- script is the path within the container to the SQL script you want to execute.
For example:
$ docker cp ./script.sql database:/tmp
$ docker exec -it database /bin/bash
$ psql -U admin -d shop < /tmp/script.sql
These commands will, in order:
- 1. Copy the local file named script.sql into the /tmp directory of the container named database.
- 2. Start an interactive shell session by executing the bash command within the database container.
- 3. Execute the script as the admin user against the database named shop.
If you want to learn more about these commands, you can learn our articles on how to copy files to a Docker container, how to run Bash in Docker, and how to run a SQL file with PSQL.
Written by
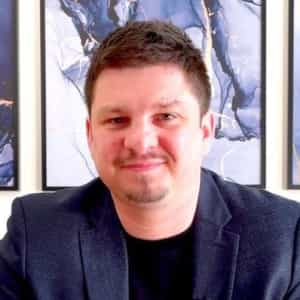
Razvan Ludosanu
Founder, learnbackend.dev
Filed Under
Related Articles
Override the Container Entrypoint With docker run
Learn how to override and customize the entrypoint of a Docker container using the docker run command.
The Dockerfile ARG Instruction
Learn how to define and set build-time variables for Docker images using the ARG instruction and the --build-arg flag.
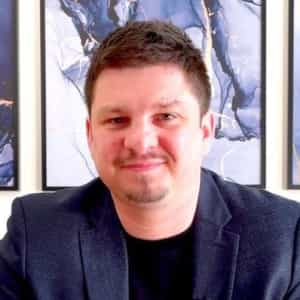
Start a Docker Container
Learn how to start a new Docker container from an image in both the foreground and the background using the docker-run command.
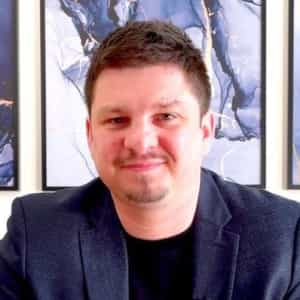
Stop All Docker Containers
How to gracefully shutdown running containers and forcefully kill unresponsive containers with signals in Docker using the docker-stop and docker-kill commands.
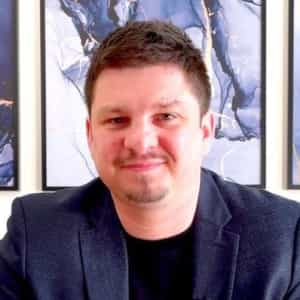
Use An .env File In Docker
Learn how to write and use .env files in Docker to populate the environment of containers on startup.
Run SSH In Docker
Learn how to launch and connect to a containerized SSH server in Docker using password-based authentication and SSH keys.
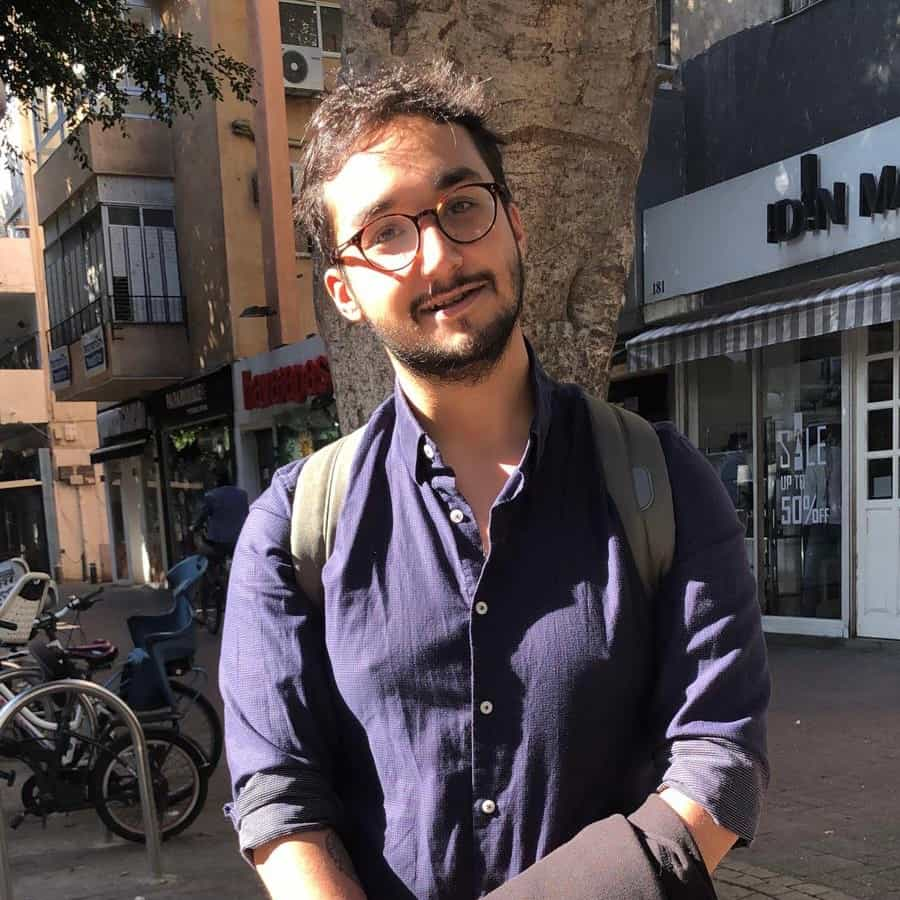
Launch MySQL Using Docker Compose
Learn how to launch a MySQL container in Docker Compose.
Execute in a Docker Container
Learn how to execute one or multiple commands in a Docker container using the docker exec command.
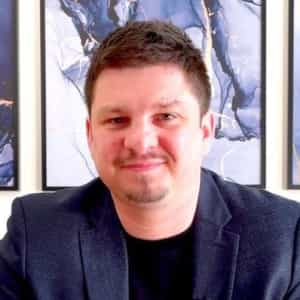
Expose Docker Container Ports
Learn how to publish and expose Docker container ports using the docker run command and Dockerfiles.
Restart Containers In Docker Compose
Learn how to restart and rebuild one or more containers in Docker Compose.
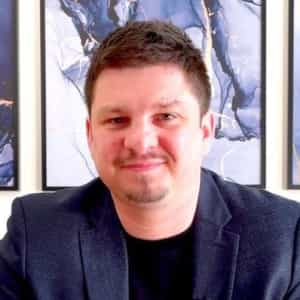
Output Logs in Docker Compose
Learn how to output, monitor, customize and filter the logs of the containers related to one or more services in Docker Compose
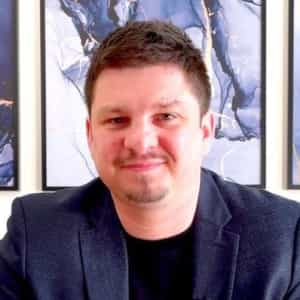
Rename A Docker Image
Learn how to rename Docker images locally and remotely using the docker tag command.