In Docker, publishing and exposing ports have different meanings.
Publishing a port refers to making the services running inside a container accessible to the outside world by mapping a container port to a host port
Exposing a port, on the other hand, simply informs Docker that the port is available for communication between containers, but does not make it accessible from outside the Docker network unless it is also published.
The short answer
To map a host port to a container port, you can use the docker run command with the -p flag (short for --publish ) as follows:
$ docker run -p <host_port>:<container_port> <image>
Run in Warp
Where:
- host_port specifies the port on the host.
- container_port specifies the port on the container.
For example, the following command will start a new container based on the nginx image and map the port 8080 of the host to the port 80 of the container:
$ docker run -p 8080:80 nginx
Run in Warp
This way, all the traffic coming on the port 8080 of the host will be automatically forwarded to the port 80 of the container.
Easily retrieve this command using Warp’s AI Command Suggestion
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestion feature:


Entering docker publish port in the AI command search will prompt a docker command that can then be quickly inserted into your shell by doing CMD+ENTER .
Publishing multiple ports at once
In Docker, it is quite common for a single container to run multiple services that require different ports to listen for incoming requests.
To publish multiple ports at once, you can repeat the -p flag several times using the following syntax:
$ docker run -p <host_port>:<container_port> [-p <host_port>:<container_port>] <image>
Run in Warp
For example, the following command will start a new container based on the nginx image and map the ports 80 , 443 , and 22 on both the host and the container:
$ docker run -p 80:80 -p 443:443 -p 22:22 nginx
Run in Warp
Publishing port ranges
As an alternative, instead of repeating the -p flag multiple times, you can publish port ranges using the following syntax:
$ docker run -p <start_port_host>-<end_port_host>:<container_start_port>-<container_end_port> <image>
Run in Warp
For example, the following command will map all the ports ranging from 8000 to 8002 on the host to each of the ports ranging from 3000 to 3002 on the container:
$ docker run -p 8000-8002:3000-3002 my-api
Run in Warp
Note that this command is equivalent to the following one:
$ docker run -p 8000:3000 -p 8001:3001 -p 8002:3002 my-api
Run in Warp
Publishing the first available host port
Port ranges can also be used to assign the first port available on the host to a specific port on the container using the following syntax:
$ docker run -p <start_port_host>-<end_port_host>:<container_port> <image>
Run in Warp
For example, the following command will test all the ports ranging from 8000 to 8010 on the host and map the first available one to the port 3000 on the container:
$ docker run -p 8000-8010:3000 my-api
Run in Warp
Publishing ports with TCP and UDP
By default, all the ports published through the -p flag use the TCP protocol, which provides a reliable and ordered delivery of data.
To publish a port with a different network communication protocol such as UDP, which prioritizes speed over perfect reliability, you can use the following syntax:
$ docker run -p <host_port>:<container_port>/<protocol> <image>
Run in Warp
For example, the following command will publish the port 80 using the UDP protocol and the port 443 using the TCP protocol:
$ docker run -p 80:80/udp -p 443:443/tcp node-server
Run in Warp
Exposing ports in a Dockerfile
To expose one or more ports in a Dockerfile, you can use the EXPOSE instruction as follows:
EXPOSE <port> [<port>/<protocol> …]
Run in Warp
Where:
- port is the port number.
- [port;/protocol] is an optional list of ports and protocols, where protocol is one of tcp or udp .
For example, the following Dockerfile exposes the UDP port 80 and the TCP port 443 :
EXPOSE 80/udp 443
Run in Warp
Note that, by default, the TCP protocol is assumed if unspecified.
Exposing port ranges via a Dockerfile
To expose a port range in a Dockerfile, you can use the EXPOSE instruction as follows:
EXPOSE <start_port>-<end_port>/[protocol]
Run in Warp
For example, the following Dockerfile exposes the TCP ports 8000 , 8001 and 8002 :
EXPOSE 8000-8002
Run in Warp
Exposing ports at runtime
To expose one or more port at runtime, you can use the docker run command with the --expose flag as follows:
$ docker run --expose <port> [--expose <port> …] <image>
Run in Warp
For example, the following command will start a new container based on the postgres image and expose the port 5432 :
$ docker run --expose 5432 postgres
Run in Warp
Mapping exposed ports to random host ports
When launching a container, you can map the ports exposed through the EXPOSE property or the --expose flag to random ports on the host machine using the docker run command with the -P flag (short for --publish-all ) as follows:
$ docker run -P <image>
Run in Warp
Once launched, you can find out the port mapping of your container using the docker port command as follows:
$ docker port <container>
Run in Warp
Where:
- container is the name or ID of the container.
You can learn more about networks and ports in Docker Compose by reading our other article on understanding port mapping in Docker Compose and creating networks in Docker Compose.
Written by
Emmanuel Oyibo
Filed Under
Related Articles
Override the Container Entrypoint With docker run
Learn how to override and customize the entrypoint of a Docker container using the docker run command.
The Dockerfile ARG Instruction
Learn how to define and set build-time variables for Docker images using the ARG instruction and the --build-arg flag.

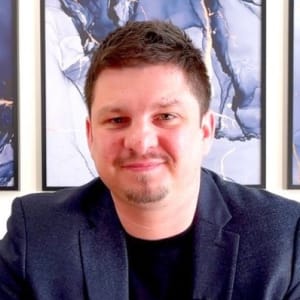
Start a Docker Container
Learn how to start a new Docker container from an image in both the foreground and the background using the docker-run command.

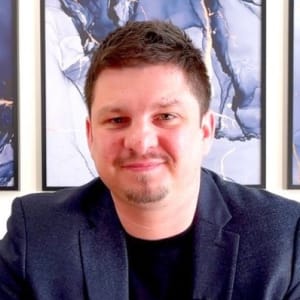
Stop All Docker Containers
How to gracefully shutdown running containers and forcefully kill unresponsive containers with signals in Docker using the docker-stop and docker-kill commands.

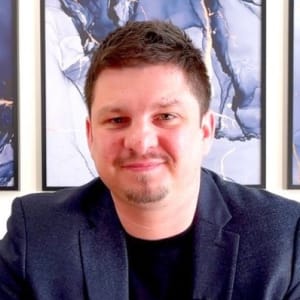
Use An .env File In Docker
Learn how to write and use .env files in Docker to populate the environment of containers on startup.
Run SSH In Docker
Learn how to launch and connect to a containerized SSH server in Docker using password-based authentication and SSH keys.

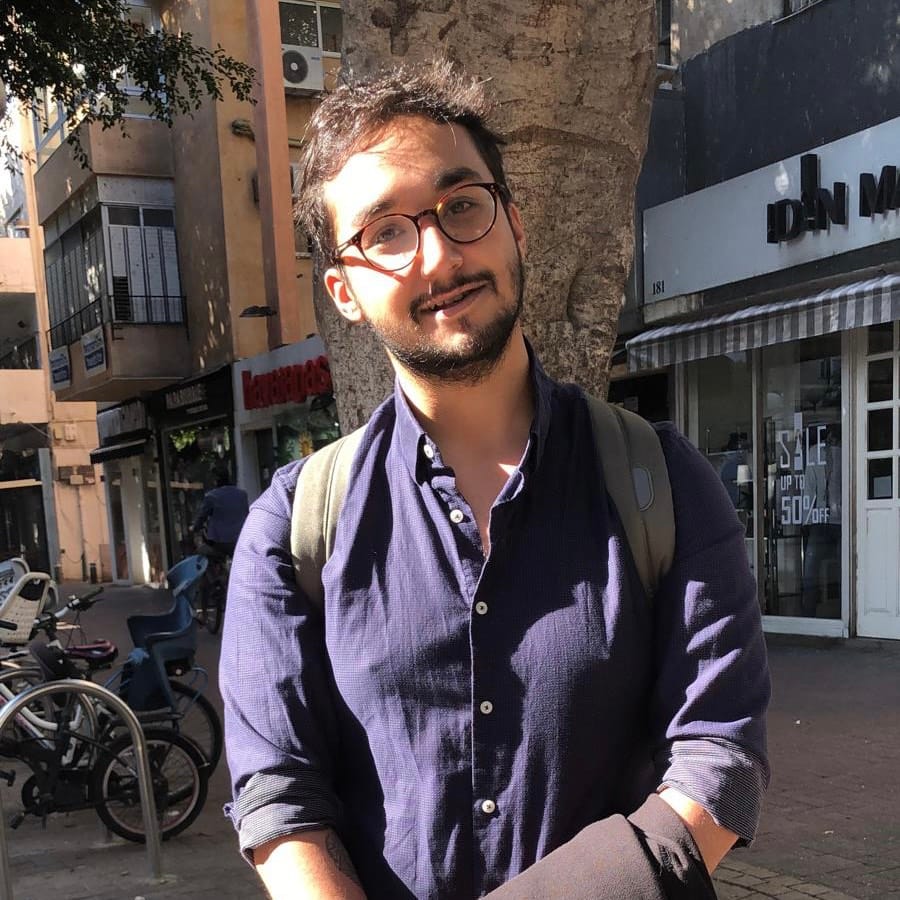
Launch MySQL Using Docker Compose
Learn how to launch a MySQL container in Docker Compose.
Execute in a Docker Container
Learn how to execute one or multiple commands in a Docker container using the docker exec command.

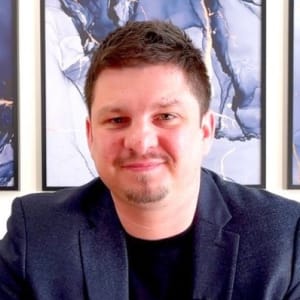
Restart Containers In Docker Compose
Learn how to restart and rebuild one or more containers in Docker Compose.

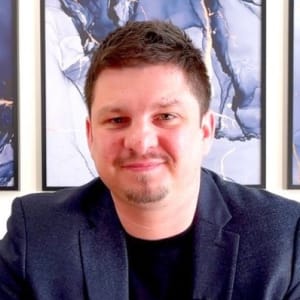
Output Logs in Docker Compose
Learn how to output, monitor, customize and filter the logs of the containers related to one or more services in Docker Compose

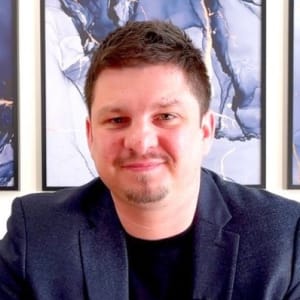
Rename A Docker Image
Learn how to rename Docker images locally and remotely using the docker tag command.
Understand healthcheck in Docker Compose
Learn how to check if a service is healthy in Docker Compose using the healthcheck property.

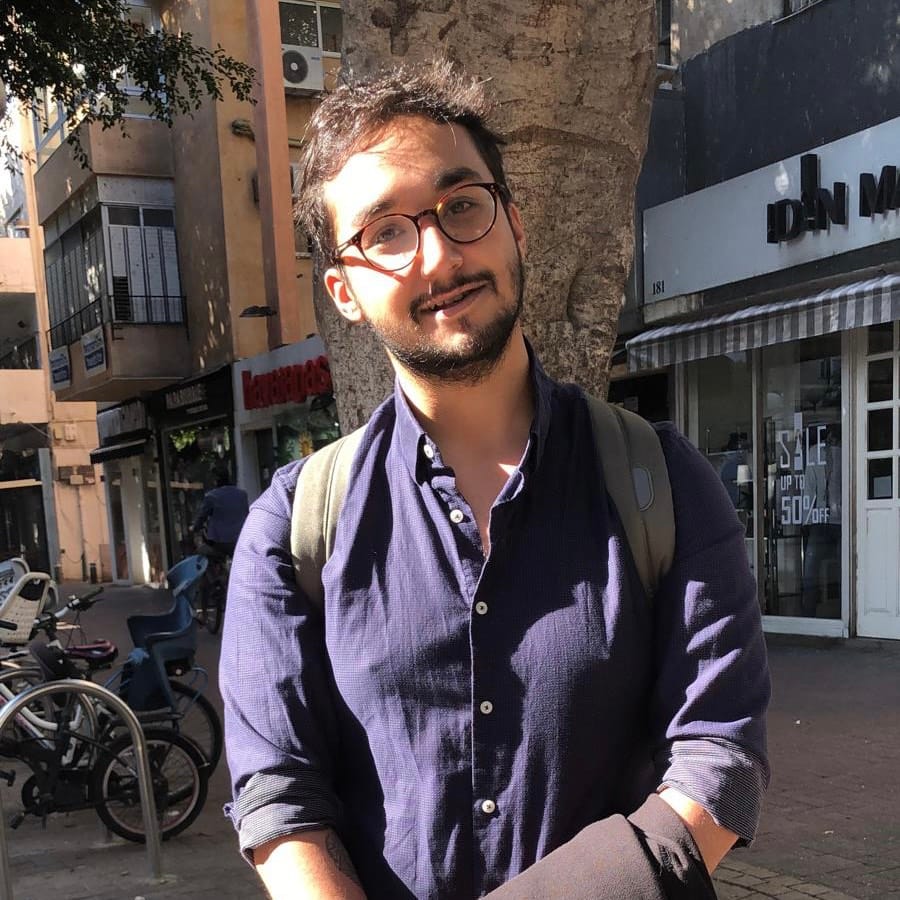