Override the Container Entrypoint With docker run
Emmanuel Oyibo
Updated: 7/24/2024
Published: 7/24/2024
In Docker, the entrypoint refers to the default executable or command that runs when a container is launched.
The short answer
To override the entrypoint of a Docker container and run another command instead on container startup, you can use the docker run command with the --entrypoint flag as follows:
$ docker run --entrypoint <command> <image>
Where:
- <command> is the command or script you want to run instead of the default entrypoint.
- <image> is the name of the Docker image you want to launch the container from.
For example, this command will replace the ubuntu image’s default entrypoint with the /bin/bash command, which gives access to an interactive Bash shell within the container:
$ docker run -it --entrypoint /bin/bash ubuntu
And this command will execute the local startup.sh script instead of the image's default entrypoint:
$ docker run --entrypoint ./startup.sh my-image
Easily retrieve this command using Warp’s AI Command Suggestions
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Suggestions feature:
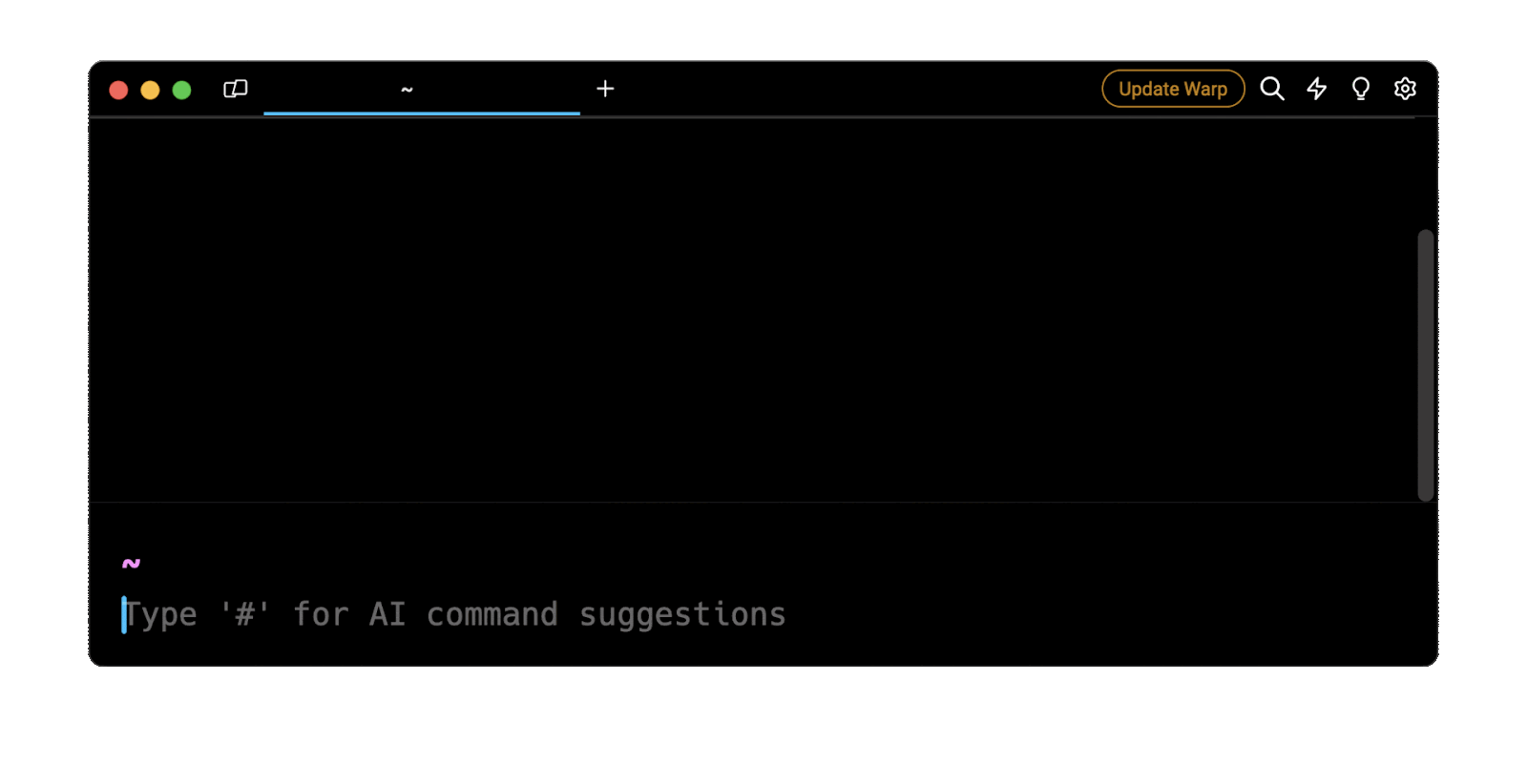
Entering docker run entrypoint in the AI Command Suggestions will prompt a docker command that can be quickly inserted into your shell by pressing CMD+ENTER.
Passing arguments to the entrypoint command
To pass one or more arguments to the specified entrypoint script or command, you can specify them after the image name in the docker run command as follows:
$ docker run --entrypoint <command> <image> <argument ...>
Where:
- <argument ...> is a list of command parameters separated by a space character.
For example, this command will launch a new container based on the ubuntu image and override the default entrypoint with the /usr/bin/python3 -m http.server command:
$ docker run --entrypoint /usr/bin/python3 ubuntu -m http.server
Combining multiple commands as an entrypoint
To combine and run multiple commands in a sequence as a single entrypoint, you can pass them as arguments of the /bin/bash -c command using the following syntax:
$ docker run --entrypoint /bin/bash <image> -c "<command_1> && <command_2> ..."
For example, this command will launch a new container based on the ubuntu image and execute the npm install and npm run test commands in a sub-shell through the /bin/bash -c command upon container startup:
$ docker run --entrypoint "/bin/bash" ubuntu -c "npm install && npm run test"
You can learn more about executing commands in a Docker container with Bash by reading our other article on how to run Bash in Docker.
Troubleshooting containers by delaying the execution of commands
One way to troubleshoot Docker containers, for instance to monitor its startup logs or verify the proper execution of specific processes within the container, you can pause the container's execution for a certain amount of seconds using the /bin/sleep command follows:
$ docker run --entrypoint "/bin/sleep" <image> <seconds>
Where:
- <seconds> is the number of seconds you want to pause the container for.
For example, this command will pause the container's execution for 20 seconds before exiting:
$ docker run --entrypoint "/bin/sleep" ubuntu 20
Executing commands as another user
By default, all the commands executed within a Docker container are executed with superuser privileges.
To execute commands as another user instead of the default root user, you can use the docker run command with the --user flag as follows:
$ docker run --entrypoint <command> --user <username> <image>
Where:
- username is the name of the user to execute the specified command as.
For example, this command will override the default entrypoint and execute the whoami command as the www-data user on container startup:
$ docker run --entrypoint "/bin/bash" --user www-data ubuntu -c "whoami"
However, note that in order to work, the specified user account must first be defined within the container's OS.
You can learn more about this by reading our other article on how to create a new user in Linux.
Changing the default working directory
To ensure that the specified entrypoint command is executed at the right location in the filesystem, you can use the docker run command with the --workdir flag as follows:
$ docker run --entrypoint <command> --workdir <directory> <image> <argument ...>
Where:
- <directory> is the absolute path to the directory the specified command will be executed in.
For example, this command will execute the pwd command within the /app directory upon container startup:
$ docker run --entrypoint "/bin/bash" --workdir /app ubuntu -c "pwd"
Mounting a local directory
It may sometimes happen that your entrypoint commands or scripts require specific files present on your local machine to run properly.
To allow the specified entrypoint to access the files located on your machine from within the container, you can create a bind mount using the -v flag as follows:
$ docker run --entrypoint <command> -v <host_directory>:<container_directory> <image>
Where:
- <host_directory> is the absolute or relative path of the bind mount on your local machine.
- <container_directory> is the absolute path of the file or directory within the container.
For example, this command will map the local /home/johndoe/website directory to the container's /app directory and execute the npm run start command upon container startup:
$ docker run --entrypoint "npm run start" -v /home/johndoe/website:/app website
You can learn more about bind mounts by reading our other article on how to mount files and directories in a Docker container.
Overriding environment variables
To set or override environment variables, you can use the docker run command with the -e flag as follows:
$ docker run --entrypoint <command> -e <variable>=<value> <image>
Where:
- <variable> is the name of the environment variable you want to set.
- <value> is the value of the environment variable.
Note that you can use the -e flag multiple times to set multiple variables.
For example, this command will set the DB_NAME environment variable to development and execute the startup.sh script upon container startup:
$ docker run --entrypoint ./startup.sh -e DB_NAME=development website
Written by
Emmanuel Oyibo
Filed Under
Related Articles
The Dockerfile ARG Instruction
Learn how to define and set build-time variables for Docker images using the ARG instruction and the --build-arg flag.
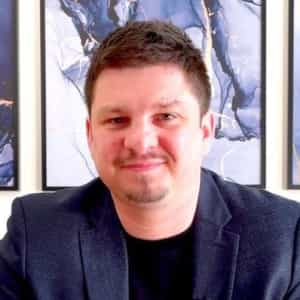
Start a Docker Container
Learn how to start a new Docker container from an image in both the foreground and the background using the docker-run command.
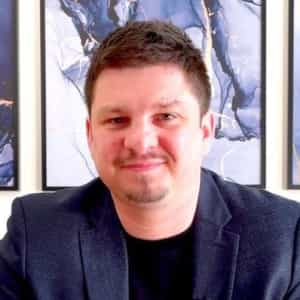
Stop All Docker Containers
How to gracefully shutdown running containers and forcefully kill unresponsive containers with signals in Docker using the docker-stop and docker-kill commands.
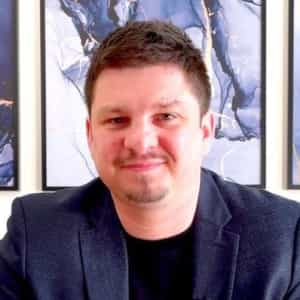
Use An .env File In Docker
Learn how to write and use .env files in Docker to populate the environment of containers on startup.
Run SSH In Docker
Learn how to launch and connect to a containerized SSH server in Docker using password-based authentication and SSH keys.
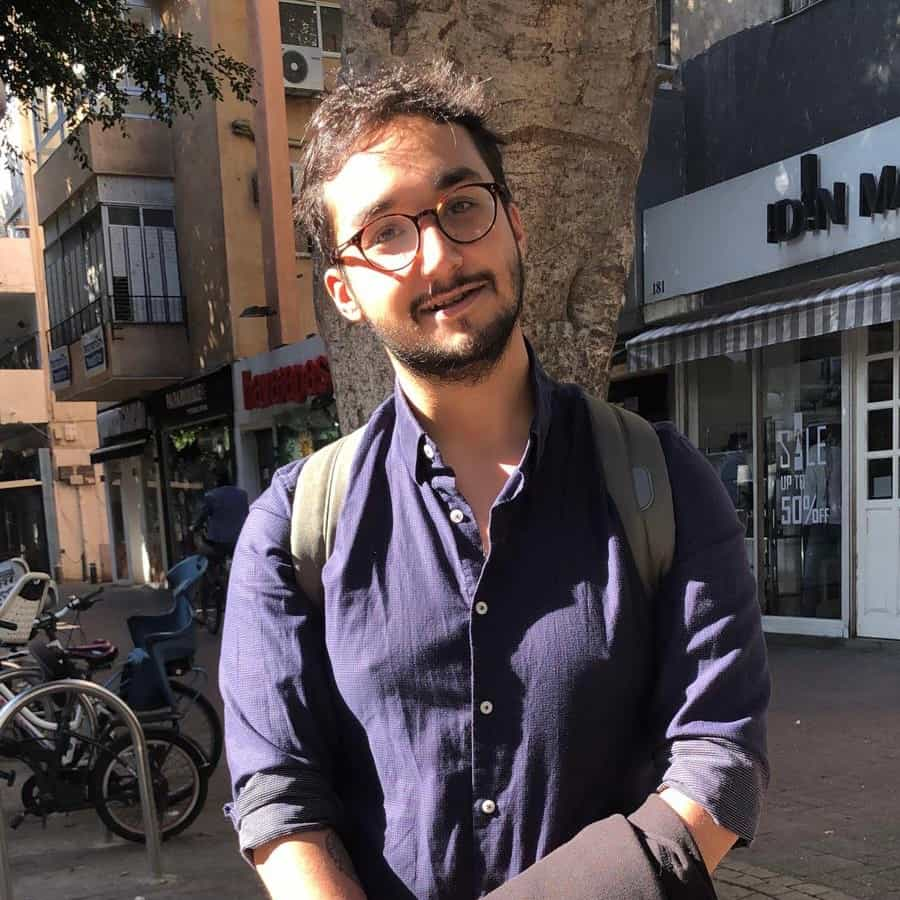
Launch MySQL Using Docker Compose
Learn how to launch a MySQL container in Docker Compose.
Execute in a Docker Container
Learn how to execute one or multiple commands in a Docker container using the docker exec command.
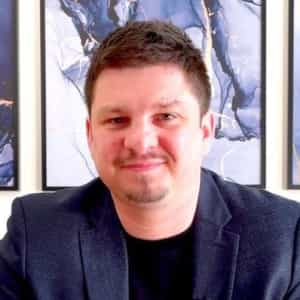
Expose Docker Container Ports
Learn how to publish and expose Docker container ports using the docker run command and Dockerfiles.
Restart Containers In Docker Compose
Learn how to restart and rebuild one or more containers in Docker Compose.
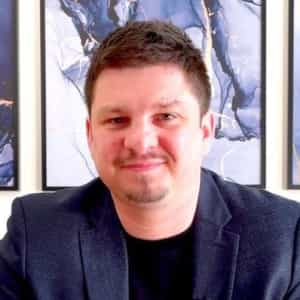
Output Logs in Docker Compose
Learn how to output, monitor, customize and filter the logs of the containers related to one or more services in Docker Compose
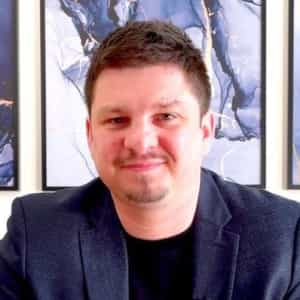
Rename A Docker Image
Learn how to rename Docker images locally and remotely using the docker tag command.
Understand healthcheck in Docker Compose
Learn how to check if a service is healthy in Docker Compose using the healthcheck property.
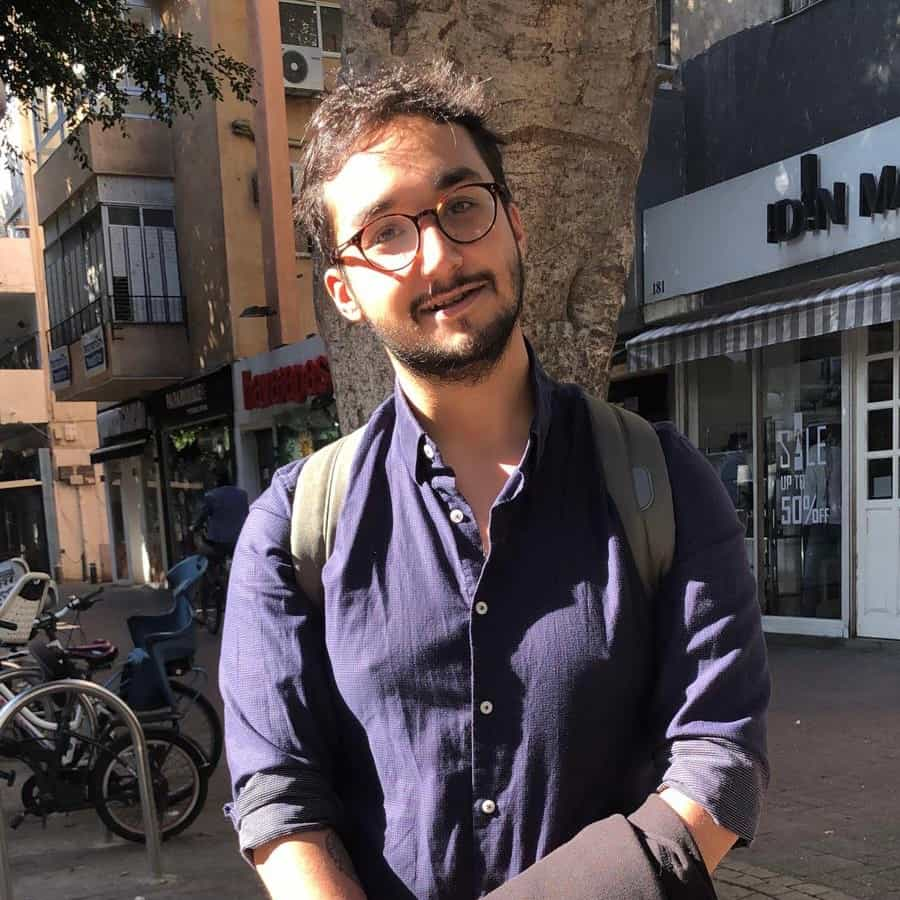