Basic Access Authentication is an HTTP authentication scheme, which consists in a client providing a username and a password when making a request to a server, to prove who they claim to be in order to access protected resources. Note that performing Basic Access Authentication with cURL differs from the idea of authorization in the sense that the latter is performed by the server in order to determine users' access rights - i.e. authorization is what happens after authentication.
The short answer
To perform Basic Access Authentication with cURL, you can use the -u option flag (short for --user) as follows:
$ curl -u username:password url
Where the username and the password are separated by a colon character (:).
Alternatively, if you only specify the username, cURL will prompt you for a password:
$ curl -u username url
Using this command inserts an “Authorization” header under the hood
cURL will encode the username:password string using the Base64URL encoding scheme and include this value in the Basic authorization header of the HTTP request. For example, the johndoe:password string will be converted by cURL into the following HTTP header:
Authorization: Basic am9obmRvZTpwYXNzd29yZA==
Remind yourself of the syntax using AI Command Search
If you’re using Warp as your terminal, you can easily retrieve this command using the Warp AI Command Search feature:
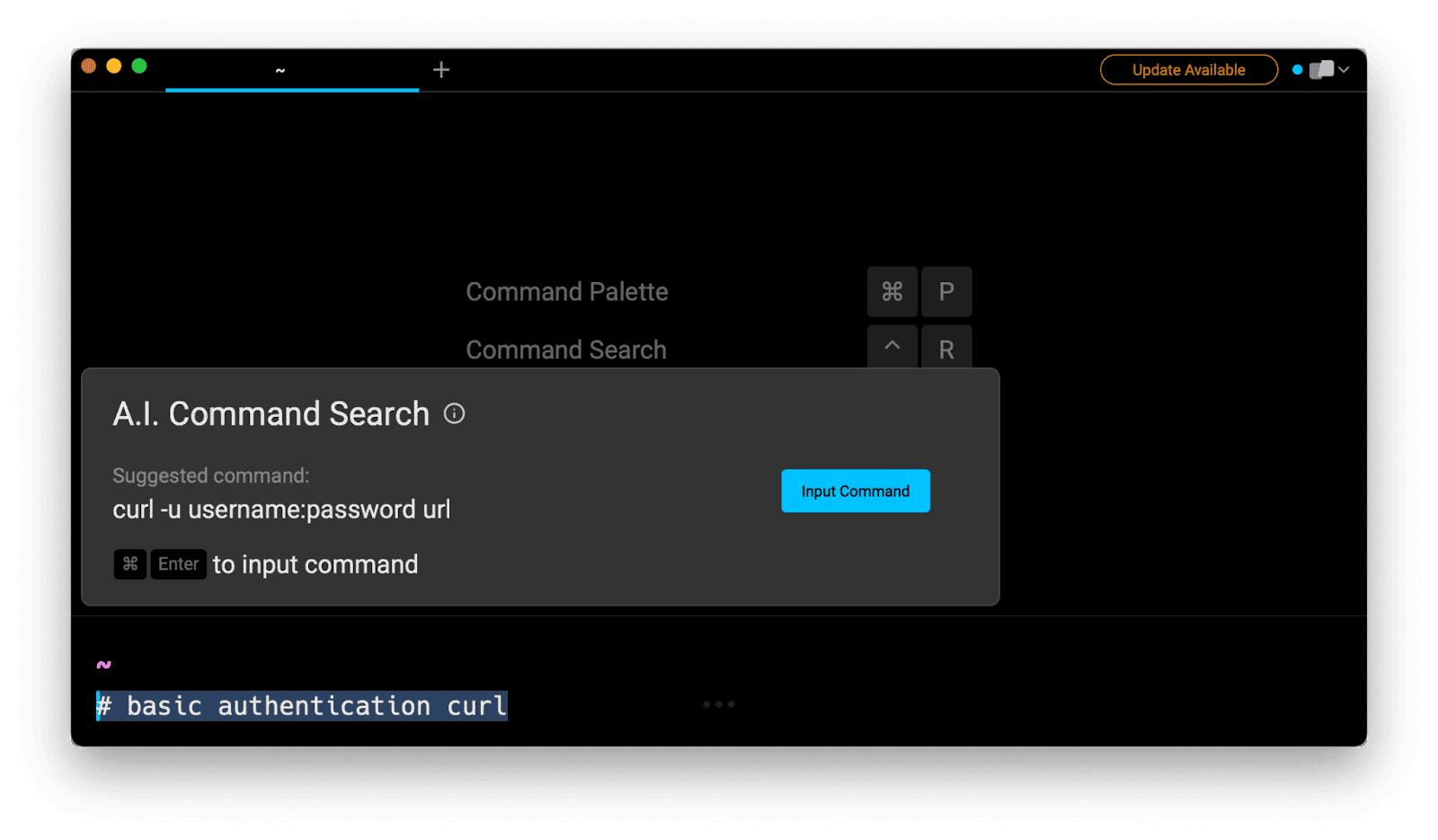
Entering basic authentication curl in the AI Command Search prompt results in exactly curl -u username:password url, which you can then quickly insert into your shell by doing CMD+ENTER.
Escaping special characters in curl (such as your password)
When using cURL for authentication, you may need to escape certain characters in your username or password.
To escape special characters, you can either use a backslash character ().
$ curl -u johndoe:h\&llo https://example.com
Or you can wrap your string in single quotes, which will cause all special characters to lose their meaning and prevent the shell from performing expansions.
$ curl -u johndoe:'h&llo' https://example.com
Here are some characters that should be escaped:
- Colon (:): the colon is used to separate the username and the password; note that this character shouldn't exist in your username, and should be escaped if it exists in your password.
- Ampersand (&): the ampersand is used by the shell to send a process to the background.
- Percent (%): the percent sign is used to encode special characters in URLs, which may cause encoding errors.
- Space: the space character is used by the shell to separate command-line arguments and options.
Use HTTPS (not HTTP) with your curl requests
Generally speaking, it is never a good idea to pass your credentials in clear text over the network using an unsecured protocol such as HTTP.
When available, you should always use the HTTPS endpoint of the service you are trying to authenticate to, by specifying the https scheme in the target URL as follow:
$ curl -u username:password https://example.com
This will add a strong layer of encryption on top of HTTP that guarantees that your credentials are safe even if they were to fall into the wrong hands.
Secure your curl credentials in a .netrc file
In general, performing an authentication by typing your credentials in clear text in the command-line constitutes a significant security risk.
The reason for that lies in the fact that, just like your browser saves the searches you perform, the shell keeps an internal history list of all the commands you run.
These commands are temporarily stored in the RAM until you log out of your current shell session, which will cause the history list to be physically written to the disk in a file located in your home directory (e.g. .bash_history for Bash, .zsh_history for ZSH, etc).
Because of that, other users registered on the system might be able to access this file and steal your credentials.
You can of course clear specific entries of the history before it is written to the disk using the history command:
$ history -d entry_number
However, a better way to secure your credentials is to retrieve them from a file only you can access.
The .netrc file
In order to avoid passing your credentials in clear text to the cURL command, you can store them in a file named .netrc located in your home directory:
default
login
password
For example:
default
login [email protected]
password h3lloJ0hn
And then use the -n option flag (short for --netrc) to perform an authentication:
$ curl -n url
Note that if you want to keep this file in another directory, you can use the --netrc-file option flag instead to specificity its path:
$ curl --netrc-file path/to/file url
For obvious security reasons, this file should only be readable and writable by you, which can be achieved using the following chmod command:
$ chmod 600 ~/.netrc
You can learn more about changing the access rights and ownership of files on Linux by reading our articles on the chmod command and the chown command.
Written by
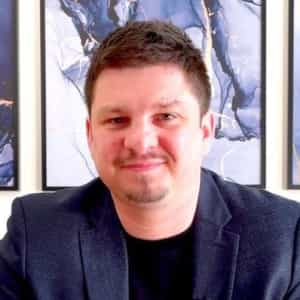
Razvan Ludosanu
Founder, learnbackend.dev
Filed Under
Related Articles
List Open Ports in Linux
Learn how to output the list of open TCP and UDP ports in Linux, as well as their IP addresses and ports using the netstat command.
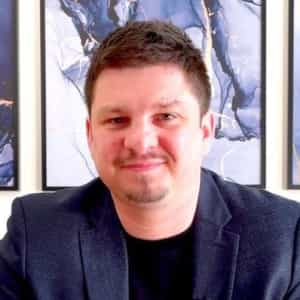
Count Files in Linux
Learn how to count files and folders contained in directories and subdirectories in Linux using the ls, find, and wc commands.
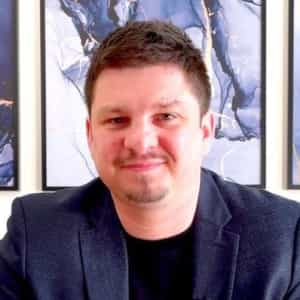
How to Check the Size of Folders in Linux
Learn how to output the size of directories and subdirectories in a human-readable format in Linux and macOS using the du command.
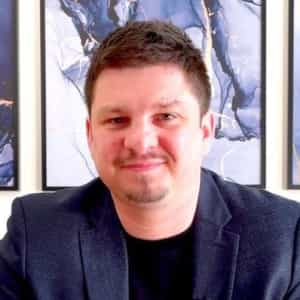
Linux Chmod Command
Understand how to use chmod to change the permissions of files and directories. See examples with various chmod options.
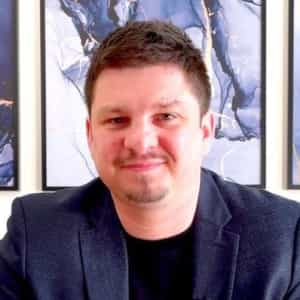
POST JSON Data With Curl
How to send valid HTTP POST requests with JSON data payloads using the curl command and how to avoid common syntax pitfalls. Also, how to solve the HTTP 405 error code.
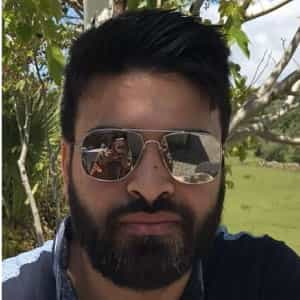
Format Command Output In Linux
Learn how to filter and format the content of files and the output of commands in Linux using the awk command.
Create Groups In Linux
Learn how to manually and automatically create and list groups in Linux.
Switch Users In Linux
Learn how to switch between users, log in as another user, and execute commands as another user in Linux.
Remover Users in Linux
Learn how to remove local and remote user accounts and associated groups and files in Linux using the userdel and deluser commands.
Delete Files In Linux
Learn how to selectively delete files in Linux based on patterns and properties using the rm command.
Find Files In Linux
Learn how to find and filter files in Linux by owner, size, date, type and content using the find command.
Copy Files In Linux
Learn how to safely and recursively copy one or more files locally and remotely in Linux using the cp and scp command.